Table of Contents
- Operators
- C# Operators
- Types of Operators
- References
- Conclusion
Operators
So In this chapter we are going to discuss about C# operators in detail but going to know about topic let’s discuss what is operator & why we use it.
Operators are used in any programming language to perform operations on variables and values.
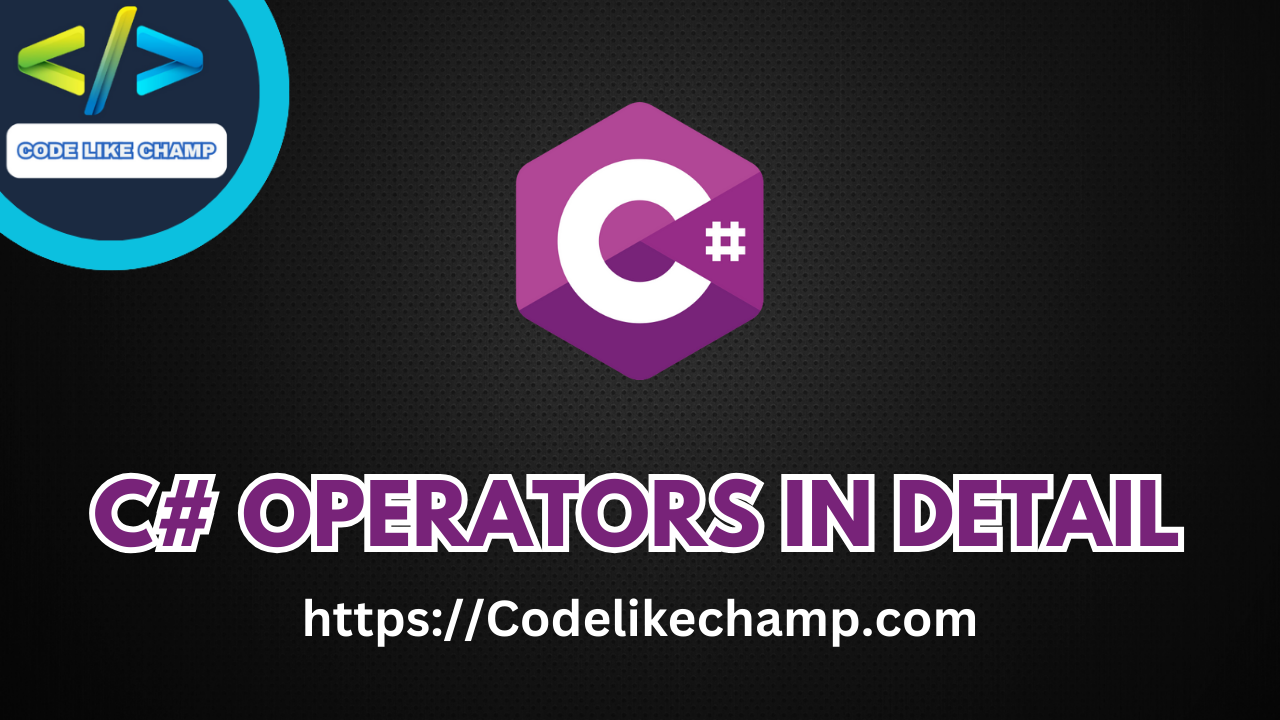
C# Operators
Now there are a lots of basic C# Operators which are used to perform basic operation on variable & values such as addition , subtraction , multiplication and much more!
Types of Operators
There are a lot’s of types of C# Operators see below the list of these operator we will discuss each of them.
Arithmetic Operators
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
Code
int num1 = 10; int num2 = 5; int sum = num1 + num2; // Addition int difference = num1 - num2; // Subtraction int product = num1 * num2; // Multiplication int quotient = num1 / num2; // Division int remainder = num1 % num2; // Modulus
Relational Operators
- Equality (==)
- Inequality (!=)
- Greater Than (>)
- Less Than (<)
- Greater Than or Equal To (>=)
- Less Than or Equal To (<=)
Code
int a = 5; int b = 7; bool isEqual = (a == b); // Equality bool isNotEqual = (a != b); // Inequality bool isGreaterThan = (a > b); // Greater Than bool isLessThan = (a < b); // Less Than bool isGreaterOrEqual = (a >= b); // Greater Than or Equal To bool isLessOrEqual = (a <= b); // Less Than or Equal To
Logical Operators
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
Code
bool x = true; bool y = false; bool resultAnd = x && y; // Logical AND bool resultOr = x || y; // Logical OR bool resultNotX = !x; // Logical NOT bool resultNotY = !y;
Bitwise Operators
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise NOT (~)
- Left Shift (<<)
- Right Shift (>>)
Code
int m = 5; // 0101 in binary int n = 3; // 0011 in binary int resultAnd = m & n; // Bitwise AND (0001) int resultOr = m | n; // Bitwise OR (0111) int resultXor = m ^ n; // Bitwise XOR (0110) int resultNotM = ~m; // Bitwise NOT (11111010) int leftShifted = m << 1; // Left Shift (1010, equivalent to multiplying by 2) int rightShifted = m >> 1; // Right Shift (0010, equivalent to dividing by 2)
Assignment Operators
- Assignment (=)
- Addition Assignment (+=)
- Subtraction Assignment (-=)
- Multiplication Assignment (*=)
- Division Assignment (/=)
- Modulus Assignment (%=)
- Bitwise AND Assignment (&=)
- Bitwise OR Assignment (|=)
- Bitwise XOR Assignment (^=)
- Left Shift Assignment (<<=)
- Right Shift Assignment (>>=)
Code
int num = 10; num += 5; // Addition Assignment (num = num + 5) num -= 3; // Subtraction Assignment (num = num - 3) num *= 2; // Multiplication Assignment (num = num * 2) num /= 4; // Division Assignment (num = num / 4) num %= 3; // Modulus Assignment (num = num % 3) num &= 1; // Bitwise AND Assignment (num = num & 1) num |= 8; // Bitwise OR Assignment (num = num | 8) num ^= 6; // Bitwise XOR Assignment (num = num ^ 6) num <<= 2; // Left Shift Assignment (num = num << 2) num >>= 1; // Right Shift Assignment (num = num >> 1)
Conditional Operator
- Conditional (Ternary) Operator (?:)
Code
int age = 20; string result = (age >= 18) ? "Adult" : "Minor";
Type Cast Operator
- Explicit Type Cast (cast_type)
- as Operator (as)
Code
double doubleValue = 10.5; int intValue = (int)doubleValue; // Explicit Type Cast object obj = "Hello"; string str = obj as string; // as Operator
Null Coalescing Operator
- Null Coalescing Operator (??)
Code
string name = null; string result = name ?? "John"; // If name is null, use "John" as the default
Member Access Operator
- Member Access (.)
Code
public class Person { public string Name { get; set; } } Person person = new Person(); person.Name = "Alice"; string name = person.Name; // Member Access
Indexer Access Operator
- Indexer Access ([])
Code
public class MyCollection { private string[] data = { "A", "B", "C" }; public string this[int index] { get { return data[index]; } set { data[index] = value; } } } MyCollection collection = new MyCollection(); string value = collection[1]; // Indexer Access
Other Operators
- is Operator (is)
- sizeof Operator (sizeof)
- typeof Operator (typeof)
Code
string text = "Hello"; bool isString = text is string; // is Operator int size = sizeof(int); // sizeof Operator Type type = typeof(string); // typeof Operator
References
- Microsoft C# Documentation: https://docs.microsoft.com/en-us/dotnet/csharp/
- C# Operators (C# Programming Guide): https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/statements-expressions-operators/operators
Conclusion
In conclusion, C# operators are fundamental elements of the language that enable developers to perform various operations on data. They range from basic arithmetic and logical operators to more advanced bitwise and conditional operators. Understanding how to use and manipulate operators efficiently is crucial for writing clean and efficient C# code. By mastering these operators, developers can harness the full power of the language and build robust software applications.
Website Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin
Youtube channel Link: Subscribe my Channel
Facebook Group: Join our Facebook Group