Before discussing data types in Java, you should be aware of the word datatype. So what is a data type? It is the type of data that a variable can store in the form of value. It can be of any kind, i.e. numerical data, textual data, character data etc. I hope you got it. If you still have any queries related to datatypes, comment below. Now let’s discuss datatypes in Java.
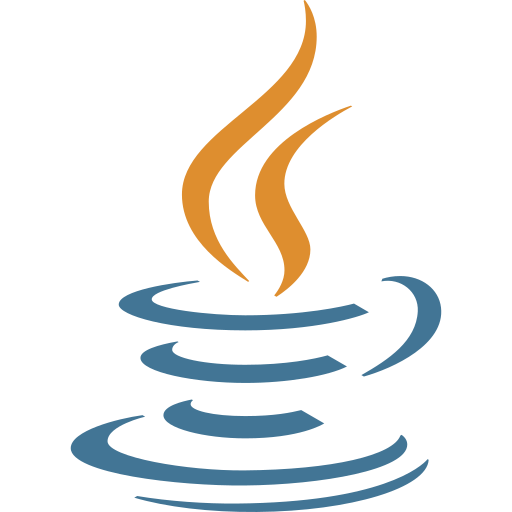
Data Types in Java
In java data types are divided into two groups:
1-Primitive data types
2-Non-primitive data types
Primitive data types | Non-primitive data types |
byte | string |
float | arrays |
int | classes |
double | |
char | |
long | |
boolean | |
short |
Declaring variable with datatypes in java with output
public class Main { public static void main(String[] args) { int myNum = 5; // integer (whole number) float myFloatNum = 5.99f; // floating point number char myLetter = 'D'; // character boolean myBool = true; // boolean String myText = "Codelikechamp"; // String System.out.println(myNum); System.out.println(myFloatNum); System.out.println(myLetter); System.out.println(myBool); System.out.println(myText); } }
Output
5 5.99 D true Codelikechamp

Primitive Data Types in JAVA
A primitive data type is one that specifies the size and type of variable values, which means what kind of data can variable can store, and it has no additional methods.
There are a total of eight primitive data types in Java:
Data Type | Size | Description |
byte | 1 bytes | Stores whole numbers from -128 to 127 |
short | 2 bytes | Stores whole numbers from -32,768 to 32,767 |
int | 4 bytes | Stores whole numbers from -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits |
double | 8 bytes | Stores fractional numbers. Sufficient for storing 15 decimal digits |
boolean | 1 bit | Stores true or false values |
char | 2 bytes | Stores a single character/letter or ASCII values |
1-Numbers(Primitive data types) Data types:
Basically java contain two types of numeric data types:
a-Integer types
These type of datatypes can stores whole numbers, positive or negative (such as 123 or -456), without decimals. Valid types are byte, short, int and long. Which type you should use, depends on the numeric value.
Byte
public class Main { public static void main(String[] args) { byte myNum = 10; System.out.println(myNum); } }
Output
10
short
public class Main { public static void main(String[] args) { short myNum = 2000; System.out.println(myNum); } }
Output
2000
int
public class Main { public static void main(String[] args) { int myNum = 200000; System.out.println(myNum); } }
Output
200000
long
public class Main { public static void main(String[] args) { long myNum = 25000000000L; System.out.println(myNum); } }
Output
25000000000
b-floating point number types
These type of datatypes represents numbers with a fractional part, containing one or more decimals. There are two types: float and double.
float
public class Main { public static void main(String[] args) { float myNum = 3.79f; System.out.println(myNum); } }
Output
3.79
double
public class Main { public static void main(String[] args) { double myNum = 20.99d; System.out.println(myNum); } }
Output
20.99
2-Boolean DataTypes
It can store values like yes/no , on/off , 1/0 etc see code below:
public class Main { public static void main(String[] args) { boolean isJavaFun = true; boolean isjavascriptFun = false; System.out.println(isJavaFun); System.out.println(isjavascriptFun); } }
Output
true false
3-Character datatype
Character type datatype can store only one character either it can be a number or alphabets:
public class Main { public static void main(String[] args) { char myGrade = 'A'; System.out.println(myGrade); } }
Output
A
4-String datatype (Non-Primitive data types)
public class Main { public static void main(String[] args) { String a = "codeliekchamp.com"; System.out.println(a); } }
Output
codeliekchamp.com
Link:Â https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin