Before discussing data types in Python, you should be aware of the word datatype. So what is a data type? It is the type of data that a variable can store in the form of value. It can be of any kind, i.e. numerical data, textual data, character data etc. I hope you got it. If you still have any queries related to datatypes, comment below. Now let’s discuss datatypes in Python.
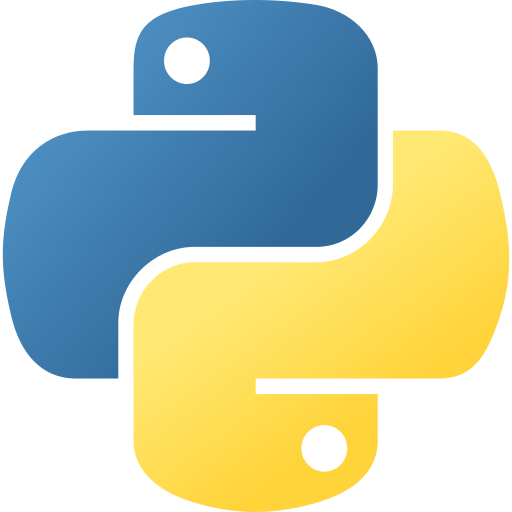
Data Types in Python
Python has the following data types built-in (i.e it is pre-defined) by default, in these categories:
TYPE OF DATA | BUILT IN DATA-TYPES |
For textual type data | Str (String) |
For numerical data | int,float,complex |
For sequence type data | list,tuple,range |
For mapping data | dict (dictionaries) |
For sets type of data | set, frozenset |
For boolean type of data | bool |
For binary type of data | bytes, bytearray, memoryview |
For none type of data | NoneType |
How to Check data types of Variable in Python
We can get type of any variable through type() function means what type of data could variable hold in the form of value. Now we make integer type variable x & assigning it value of 5 and check the type of this variable x through type() function see below.
x = 5 print(type(x))
Output
<class 'int'>
Which mean it is integer type variable.
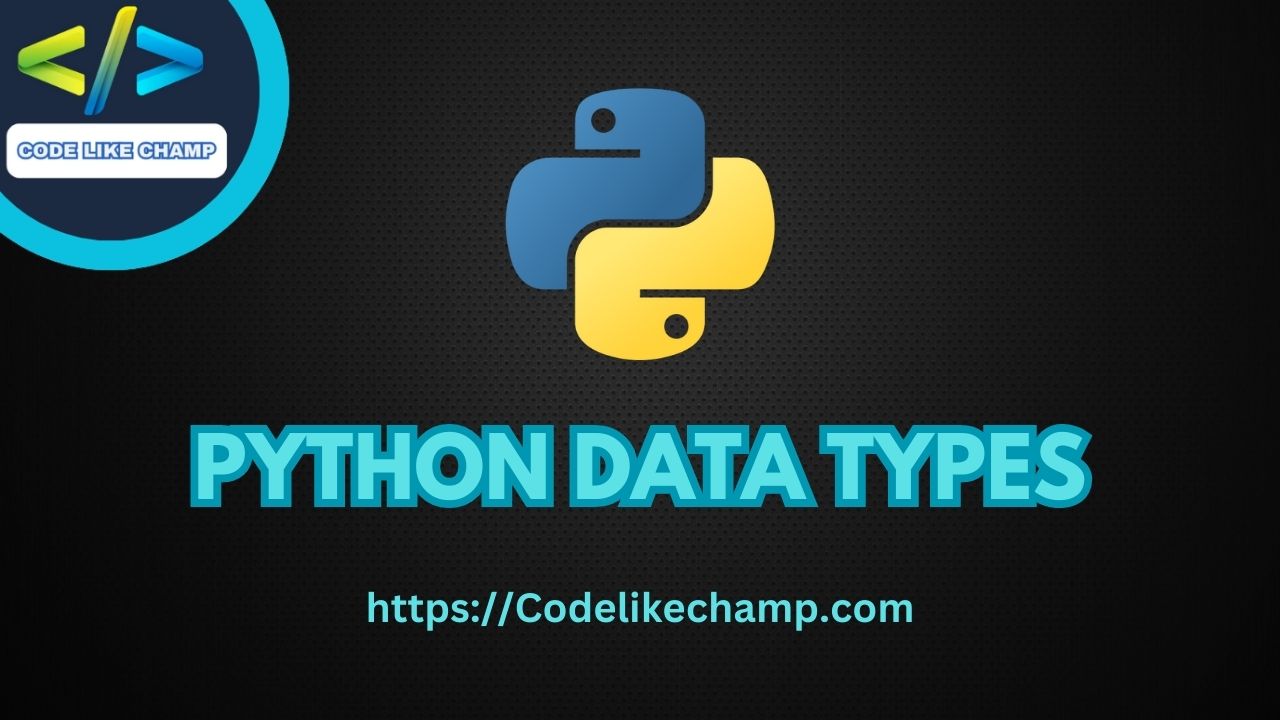
How can we set data types to Variable
In python as it is a dynamic language so when we put value in a variable so according to it value that we have give to it so the data type of particular variable is choosen look below:
For str (String)data type:
x = "Codelikechamp.com" #display x: print(x) #display the data type of x: print(type(x))
Output
Codelikechamp.com <class 'str'>
For int (numeric)data type:
x = 20 #display x: print(x) #display the data type of x: print(type(x))
Output
20 <class 'int'>
For float (numeric) & (decimal points number)data type:
x = 20.5 #display x: print(x) #display the data type of x: print(type(x))
Output
20.5 <class 'float'>
For complex (numeric)data type:
x = 1j #display x: print(x) #display the data type of x: print(type(x))
Output
lj <class 'complex'>
For list data type:
x = ["apple", "banana", "cherry"] #display x: print(x) #display the data type of x: print(type(x))
Output
['apple', 'banana', 'cherry'] <class 'list'>
For tuple data type:
x = ("apple", "banana", "cherry") #display x: print(x) #display the data type of x: print(type(x))
Output
('apple', 'banana', 'cherry') <class 'tuple'>
For range data type:
x = range(6) #display x: print(x) #display the data type of x: print(type(x))
Output
range(0, 6) <class 'range'>
For dict (dictionaries)data type:
x = {"name" : "John", "age" : 36} #display x: print(x) #display the data type of x: print(type(x))
Output
{'name': 'John', 'age': 36} <class 'dict'>
For set data type:
x = {"apple", "banana", "cherry"} #display x: print(x) #display the data type of x: print(type(x))
Output
{'banana', 'cherry', 'apple'} <class 'set'>
For frozenset data type:
x = frozenset({"apple", "banana", "cherry"}) #display x: print(x) #display the data type of x: print(type(x))
Output
frozenset({'banana', 'apple', 'cherry'}) <class 'frozenset'>
For bool data type:
x = True #display x: print(x) #display the data type of x: print(type(x))
Output
True <class 'bool'>
For bytes data type:
x = b"Hello" #display x: print(x) #display the data type of x: print(type(x))
Output
b'Hello' <class 'bytes'>
For bytearray data type:
x = bytearray(5) #display x: print(x) #display the data type of x: print(type(x))
Output
bytearray(b'\x00\x00\x00\x00\x00') <class 'bytearray'>
For memoryview data type:
x = memoryview(bytes(5)) #display x: print(x) #display the data type of x: print(type(x))
Output
<memory at 0x006F8FA0> <class 'memoryview'>
For NoneType data type:
x = None #display x: print(x) #display the data type of x: print(type(x))
Output
None <class 'NoneType'>
Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin