Now in this chapter, We will learn about Python List in detail and how can we use Python list to perform various operation on that particular list such as accessing first or last element in list etc.
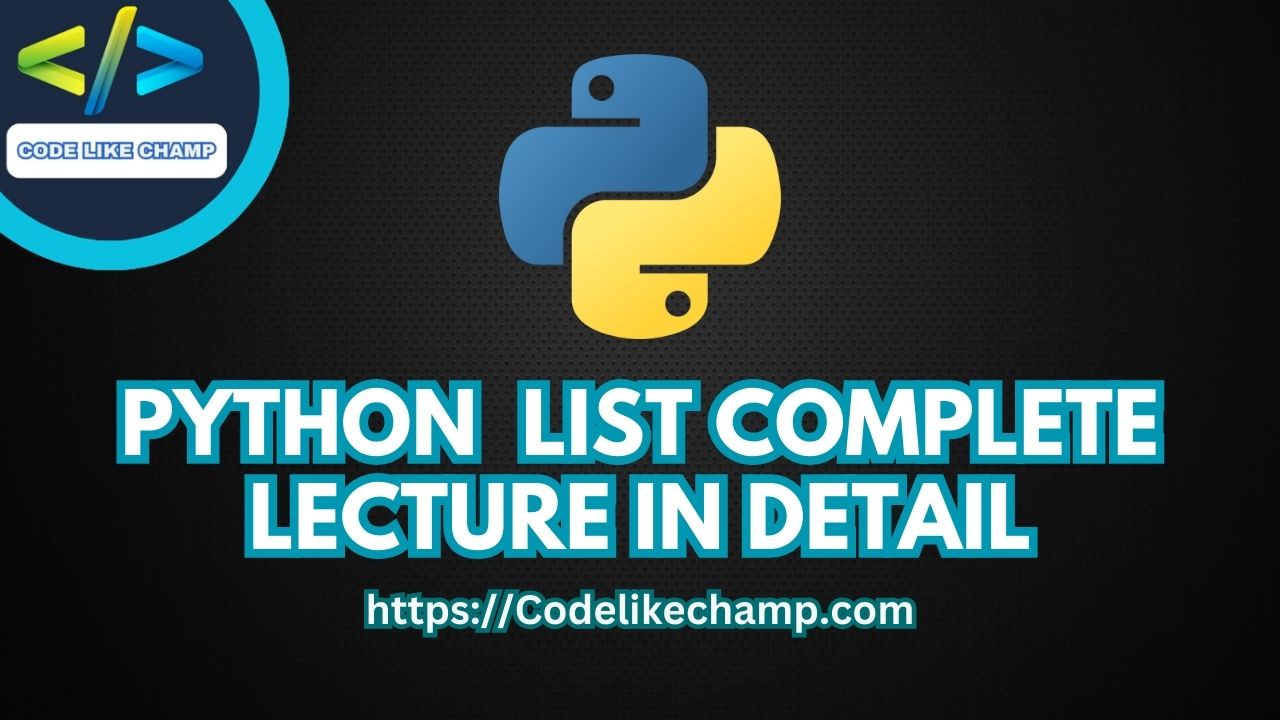
What is Python List
Python list in which we can store multiple items in a single variable which we have declared so we use this concept like in every programming languages, But in Python programming list is one of built-in data types to store multiples items in a variable as i discussed earlier the other 3 are Tuple, Set, & Dictionary which have their own purpose and benefits. We will discuss these later look below how to create a list in python.
mylist = ["C#", "PYTHON", "JAVA"] print(mylist)
Output
['C#', 'PYTHON', 'JAVA']
We have created a mylist in above python code by declaring mylist as our list variable and using square brackets putting 3 items or you can say elements in it i.e (C# , PYTHON , JAVA) & simple print list using print() function.
NOTE: That List item are in ordered, they are changeable, & they allow duplicate values, see below example for clarification.
Ordered mean: The items are in order i.e in above code C# is 1 item and PYTHON is 2 element and JAVA is 3 item/element.
Changeable mean: The items are in list can be changed, or added, and can be removed.
Duplicate means: We can create two items of same values see below example.
mylist = ["C#", "PYTHON", "JAVA", "C#", "JAVA"] print(mylist)
Output
['C#', 'PYTHON', 'JAVA', 'C#', 'JAVA']
Note: The lists are indexed
What does this mean that each item in a list have an identity mean first element in mylist is C# so it have “0” index then PYTHON is next item so it have “1” index means in a list like an array indexing is start from zeros and so on.
how to get the length of a list python
Now we can also check our List length in Python using len() function see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] print(len(mylist))
Output
3
Type of list Python
We can also check type of our list means, is it list or not, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] print(type(mylist))
Output
<class 'list'>
Python first element in list
As we discussed that list are indexed so each element can be accessed by using it index number like for first item we use 0 as indexing number etc, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] print(mylist[0]) print(mylist[1]) print(mylist[2])
Output
C# PYTHON JAVA
Python list index -1
What is negative indexing, means start indexing or you can say accessing element from end, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] print(mylist[-1])#means last element print(mylist[-2])#means second last element print(mylist[-3])#means third last element
Output
JAVA PYTHON C#
Slicing list in python
Now what is slicing, when we want to show some part of list or we tell or declare range so in return new list is create, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] print(mylist[0:2])#slice list from first element to second element excluding 3 ,4 & 5 element print(mylist[0:3])#slice list from first element to third element excluding 4 & 5 element
Output
['C#', 'PYTHON'] ['C#', 'PYTHON', 'JAVA']
NOTE: FIRST ELEMENT CAN ACCESSED BY ZERO INDEX NUMBER BECAUSE WE ARE USING LIST
By leaving out the end value in slicing, the range will go on to the end of the list, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] print(mylist[0:]) print(mylist[2:]) print(mylist[3:]) print(mylist[4:])
Output
['C#', 'PYTHON', 'JAVA', 'Scala', 'c++'] ['JAVA', 'Scala', 'c++'] ['Scala', 'c++'] ['c++']
Negative Slicing in Python
Now what is Negative slicing, when we want to show part of list or we tell or declare range from end so in return new list is create, see below example for more clarification.
#Negative indexing means starting from the end of the list. mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] print(mylist[-5:-4])#This example returns the items from index -5 (included) to index -4 (excluded) print(mylist[-5:-3])#This example returns the items from index -5 (included) to index -3 (excluded) print(mylist[-5:-2])#This example returns the items from index -5 (included) to index -2 (excluded) print(mylist[-5:-1])#This example returns the items from index -5 (included) to index -1 (excluded) #Remember that the last item has the index -1,
Output
['C#'] ['C#', 'PYTHON'] ['C#', 'PYTHON', 'JAVA'] ['C#', 'PYTHON', 'JAVA', 'Scala']

Check if an item exists in a List python
As like we can check type & length of our list so we can also check if an item is present or not present in our list using in Keyword, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] if "PYTHON" in mylist: print("Yes, 'PYTHON' is in the mylist list creted by Codelikechamp.com")
Output
Yes, 'PYTHON' is in the mylist list creted by Codelikechamp.com
Change item in list python
We can also change any individual item or more than one item at a time, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] mylist[0] = "C# change" #change item no 1 C# TO "C# change" Because index no is 0 print(mylist)
Output
['C# change', 'PYTHON', 'JAVA', 'Scala', 'c++']
Replace multiple items in list python
We can also change multiple items in a list at a time with the help of slicing, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] mylist[0:1] = ["PYTHON","C#"]#CHANGE VALUE OF 1 AND 2 ELEMENT OR ITEM IN A LIST print(mylist)
Output
['PYTHON', 'C#', 'PYTHON', 'JAVA', 'Scala', 'c++']
Insert item in List python
To insert a new item in a list, without replacing or changing any of the existing values/elements, we can use the insert()
method.
The insert()
method inserts an item at the specified index, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] mylist.insert(2, "javascript")#insert javascript at java place because index no is 2 print(mylist)
Output
['C#', 'PYTHON', 'javascript', 'JAVA', 'Scala', 'c++']
Add items to a List in python
To insert a new item in a list at last, we can use the append()
method, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA","Scala","c++"] mylist.append("javascript") print(mylist)
Output
['C#', 'PYTHON', 'JAVA', 'Scala', 'c++', 'javascript']
Join Two Lists Python
To add list item from on to another and make new list use extend() function, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] mylist2 = ["Scala","javascript"] mylist.extend(mylist2) print(mylist)
Output
['C#', 'PYTHON', 'JAVA', 'Scala', 'javascript']
Remove Item in list python
To remove an item from list use remove() function, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] mylist.remove("JAVA")#removes java from list print(mylist) mylist.pop()#pop method delete last iem of list i.e PYTHON print(mylist) del mylist[0] #del delete specific index no item here is first item I.E C# print(mylist)
Output
['C#', 'PYTHON'] ['C#'] []
How to clear the List in Python
To Clear entire list elements use clear() function, see below example for more clarification.
mylist = ["C#", "PYTHON", "JAVA"] mylist.clear() print(mylist)
Output
[]
Loop Through a List in python
To find all items in a list we can use for loop so we can iterate through each elements in a list, see below example for more clarification.
print("using for loop") mylist = ["C#", "PYTHON", "JAVA"] for x in mylist: print("\t"+x) print("using while loop") i = 0 while i < len(mylist): print("\t"+mylist[i]) i = i + 1 print("loop through the index numbers") for i in range(len(mylist)): print("\t"+mylist[i]) print("looping using list comprehension") [print("\t"+x) for x in mylist]
Output
using for loop C# PYTHON JAVA using while loop C# PYTHON JAVA loop through the index numbers C# PYTHON JAVA looping using list comprehension C# PYTHON JAVA
How to sort list in Python
Sort list in ascending order
List objects have a sort()
method that will sort the list alphanumerically and in ascending order, see below example for more clarification.
mylist = ["C", "PYTHON", "JAVA"] mylist.sort() print(mylist) mylist2 = ["100", "50", "20"] mylist2.sort() print(mylist2)
Output
['C', 'JAVA', 'PYTHON'] ['100', '20', '50']
Sort list in descending order
If you want to sort list in descending order use keyword argument reverse=True, see below example for more clarification.
mylist = ["C", "PYTHON", "JAVA"] mylist.sort(reverse = True) print(mylist) mylist2 = ["100", "50", "20"] mylist2.sort(reverse = True) print(mylist2)
Output
['PYTHON', 'JAVA', 'C'] ['50', '20', '100']
How to reverse the order of a list in python
If you want to Reverse order of list regardless of alphabets order use reverse() method, see below example for more clarification.
mylist = ["C", "PYTHON", "JAVA"] mylist.reverse() print(mylist)
Output
['JAVA', 'PYTHON', 'C']
How to make copy of a list in python
If you want to make copy of use copy() method or by using built-in list() method, see below example for more clarification.
mylist = ["C", "PYTHON", "JAVA"] list2 = mylist.copy() list3 = list(mylist) print(mylist) print(list2) print(list3)
Output
['C', 'PYTHON', 'JAVA'] ['C', 'PYTHON', 'JAVA'] ['C', 'PYTHON', 'JAVA']
Link:Â https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin
iam Very Hardworking Stude and ima learning language’s
great how can we help you our motto is binding nation through education if you want learn about programming languages we are here to help you 24/7 just come to our site we post daily content about programing languages such as Python , C++ C# , Java etc if you have any question please ask without any hesitation.
thanks for you comment.