Introduction to Python Programs
Python is a high-level programming language known for its simplicity and readability. It is so popular and most useful programming language in the software development world due to its vast ecosystem of libraries and frameworks. In this article, we will dive into the world of Python programs, exploring various aspects, from the fundamentals to advanced use cases. Today in this article we are going to learn at least most important concept of Python programming with Python code implementation i hope you will like this article and support me.
Getting Started with Python
1. Setting Up Python
Python is pre-installed on many operating systems, but you can download and install it from the official website Python.org. We recommend using the latest version to access the latest features and security updates.
2. Writing Your First Python Program
Let’s start with the simple “Hello, World!” program:
print("Hello, World!")
Variables and Data Types
3.Declaring Variables
In Python, you can declare variables without specifying their data types explicitly. For instance:
name = "John" age = 30
4.Common Data Types
Python supports various data types, including integers, floats, strings, lists, tuples, dictionaries, and more. Here’s an example of each:
my_integer = 42 my_float = 3.14 my_string = "Hello, Python!" my_list = [1, 2, 3, 4, 5] my_tuple = (1, 2, 3) my_dict = {'name': 'Alice', 'age': 25}
Control Structures
5.Conditional Statements
Python allows you to use if, elif, and else statements for controlling program flow. Here’s an example:
x = 10 if x > 5: print("x is greater than 5") elif x == 5: print("x is equal to 5") else: print("x is less than 5")
6.Loops
Python supports both “for” and “while” loops. Here’s a “for” loop example to iterate over a list:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
Functions and Modules
7.Defining Functions
Functions in Python allow you to encapsulate a block of code and reuse it. Here’s an example:
def greet(name): return f"Hello, {name}!" message = greet("Alice") print(message)
8.Importing Modules
Python has a vast standard library, and you can import modules to extend the functionality of your programs. For instance, you can import the math module for mathematical operations:
import math result = math.sqrt(16) print(result)
File Handling
9.Reading from and Writing to Files
Python makes it easy to work with files. You can read and write text files like this:
# Writing to a file with open("my_file.txt", "w") as file: file.write("This is some text.") # Reading from a file with open("my_file.txt", "r") as file: content = file.read() print(content)
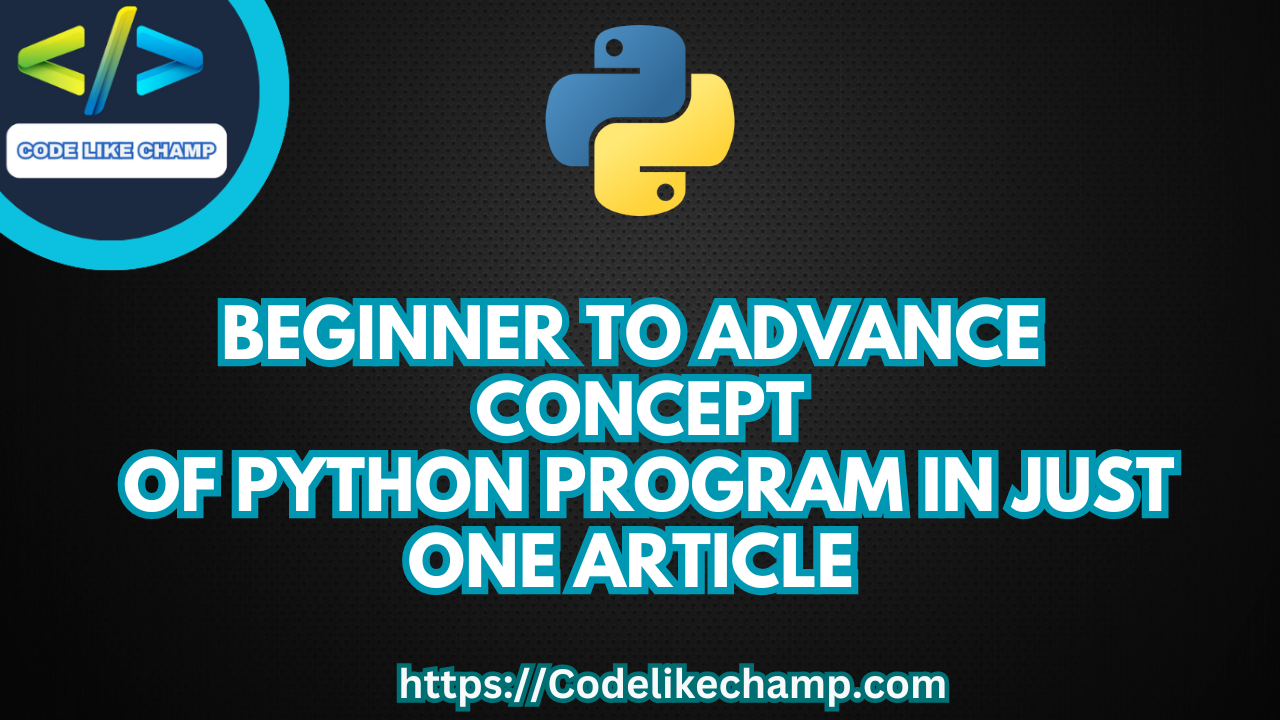
Object-Oriented Programming
10.Classes and Objects
Python is an object-oriented language, and you can create classes and objects to model real-world entities. Here’s a simple example:
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): print(f"{self.name} says Woof!") my_dog = Dog("Buddy", 3) my_dog.bark()
Exception Handling
11.Handling Exceptions
Python allows you to catch and handle exceptions using try and except blocks. For example, if you want to handle a division by zero error:
try: result = 5 / 0 except ZeroDivisionError: print("Cannot divide by zero.")
Working with Lists and Dictionaries
12.List Manipulation
Python provides various methods to manipulate lists. You can add, remove, or search for elements in a list. Here’s how to append an element to a list:
my_list = [1, 2, 3] my_list.append(4) print(my_list)
13.Dictionary Operations
Dictionaries are key-value pairs. You can add, access, and delete items from a dictionary. Here’s an example:
my_dict = {'name': 'Alice', 'age': 25} print(my_dict['name']) del my_dict['age']
Regular Expressions
14.Using Regular Expressions
Python’s re module allows you to work with regular expressions for pattern matching. Here’s an example to match an email address:
import re text = "Contact us at contact@example.com" pattern = r'\S+@\S+' matches = re.findall(pattern, text) print(matches)
Web Scraping with Python
15.Beautiful Soup for Web Scraping
Beautiful Soup is a popular Python library for web scraping. You can use it to extract data from HTML and XML documents. Here’s a simple example:
import requests from bs4 import BeautifulSoup url = 'https://example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') title = soup.title.string print(title)
Data Analysis with Pandas
16.Introduction to Pandas
Pandas is a powerful library for data manipulation and analysis. You can load, manipulate, and analyze data with ease. Here’s a basic example:
import pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) print(df)
Automation with Python
17.Automating Tasks
Python can be used for automating repetitive tasks. For example, you can create a script to rename files in a directory:
import os directory = '/path/to/directory' for filename in os.listdir(directory): if filename.endswith('.txt'): new_name = filename.replace('old_', 'new_') os.rename(os.path.join(directory, filename), os.path.join(directory, new_name))
Building Web Applications
18.Web Development with Flask
Flask is a micro web framework for Python. You can build web applications with ease. Here’s a basic example of a Flask application:
from flask import Flask app = Flask(__name) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run()
Data Visualization with Matplotlib
19.Creating Charts and Graphs
Matplotlib is a popular library for creating charts and graphs. You can visualize data effectively. Here’s a simple example:
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [10, 15, 13, 17, 20] plt.plot(x, y) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Sample Chart') plt.show()
Machine Learning with Python
20.Introduction to Machine Learning
Python is widely used in the field of machine learning. You can work with libraries like scikit-learn and TensorFlow to build and train models. Here’s a simple example:
from sklearn.linear_model import LinearRegression # Create a linear regression model model = LinearRegression() # Train the model model.fit(X, y) # Make predictions predictions = model.predict(X_test)
Conclusion
In this article, we’ve covered a wide range of topics related to Python programming. From the basics of setting up Python and writing your first program to advanced topics like web scraping, data analysis, and machine learning, Python offers a vast ecosystem and endless possibilities. With the knowledge and skills gained here, you’ll be well on your way to becoming a proficient Python programmer. If you face any issue just contact me.
Website Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin
Youtube channel Link: Subscribe my Channel
Facebook Group: Join our Facebook Group