C# Type Casting
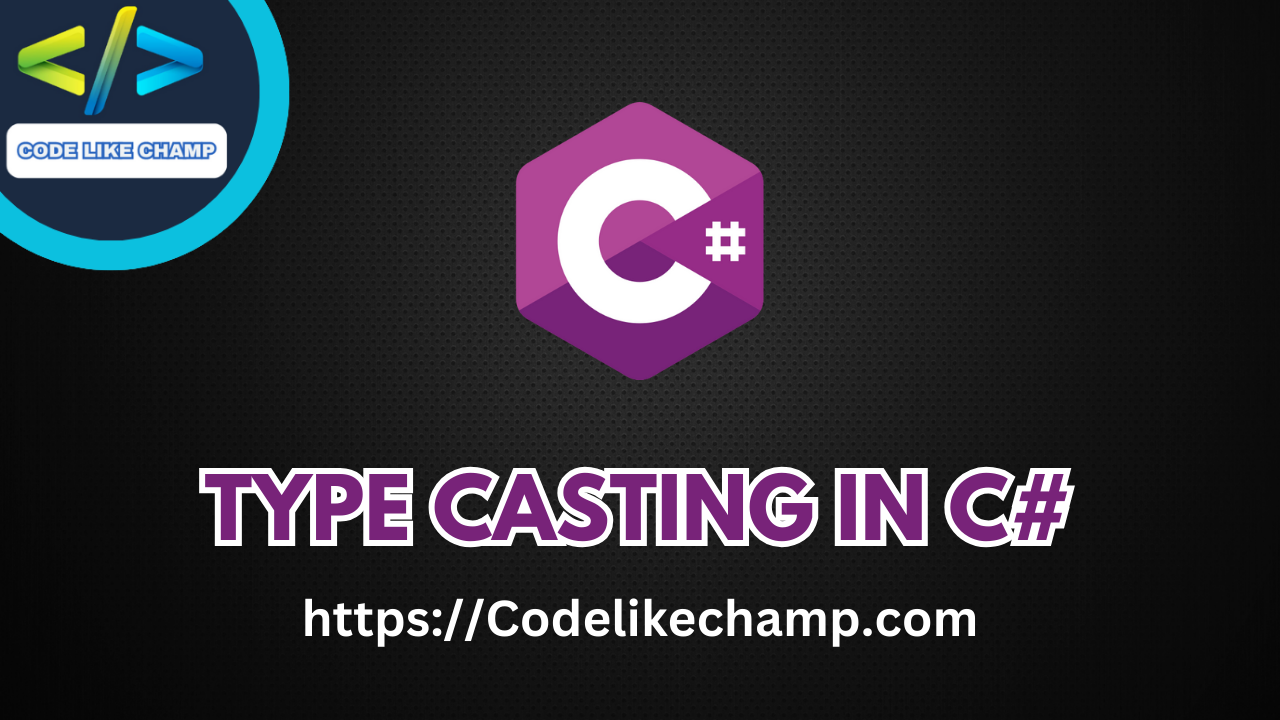
Table of Contents
- What is Type Casting?
- Type Casting in C#
- Types of Type Casting in C#
- Type Conversion Methods
- References
- Conclusion
What is Type Casting?
First let discuss what is type casting?
The meaning of Type Casting is to change one data type of a variable into another data type. Developers or programmers change data type according to their needs. Let us understand this with the help of a real practical example.
Type Casting in C#
In C#, implicit and explicit conversions are techniques used to convert one data type into another. These conversions allow you to work with different data types while maintaining type safety.
Types of Type Casting in C#
There are two types of Conversion:
- Implicit Conversion
- Explicit Conversion
Now discuss each of them:
Implicit Conversion (Widening Conversion):
Implicit conversion, also known as widening conversion. It is performed by the C# compiler automatically when it is safe to do so. It involves converting a smaller data type into a larger data type without the risk of data loss.
For example, you can implicitly convert an int datatype Variable to a double or a float to a double datatype Variable because there is no loss of precision.
int intValue = 42; double doubleValue = intValue; // Implicit conversion from int to double
Explicit Conversion (Narrowing Conversion):
Explicit conversion, also known as narrowing conversion. In this type of conversion it depends on the programmer needs to specify the conversion explicitly using casting or conversion methods. It is used when there’s a risk of data loss, and the programmer must confirm the conversion. For example, you might explicitly convert a double to an int or a float when you want to truncate the decimal part.
double doubleValue = 42.75; int intValue = (int)doubleValue; // Explicit conversion (casting) from double to int
Type Conversion Methods
Additionally, C# provides methods and properties for explicit conversion between data types, such as Convert.ToInt32(), Convert.ToDouble(), and so on:
using System; namespace MyApplication { class Program { static void Main(string[] args) { int myInt = 100; double myDouble = 6.25; bool myBool = true; Console.WriteLine(Convert.ToString(myInt)); // Convert int to string Console.WriteLine(Convert.ToDouble(myInt)); // Convert int to double Console.WriteLine(Convert.ToInt32(myDouble)); // Convert double to int Console.WriteLine(Convert.ToString(myBool)); // Convert bool to string } } }
Output:
100 100 6 True
References
- Microsoft C# Documentation:
- Microsoft’s official C# documentation provides comprehensive information on data type conversions in C#:
- C# Station:
- C# Station offers tutorials and articles on C# programming, including a section on type conversion:
- TutorialsPoint:
- TutorialsPoint provides a C# tutorial that covers type conversions:
Conclusion
In summary, implicit conversions are performed automatically by the compiler when there’s no risk of data loss, while explicit conversions require the programmer’s intervention and are used when there’s a risk of data loss or when converting between incompatible data types, I hope you understand it well.
Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin