Now before going to discuss what a C# Variable in the context of programming language. We should know what the variable word means in the context of Programming language.

Variable in General Programming language
A Variable is a particular container with a datatype and name, and a value that can be stored in it is called a variable. We can do multiple operations on it depending on their datatypes. Now, what is a data type? We shall discuss this in the next post. Now consider a Variable as a container or a thing that can store values means it can be of any datatype, i.e., it can be alphabets, it can be a string, it can be an integer, it can be a floating point number or complex number okay look here below the syntax of variable in general programming languages:
Syntax of Variable in General Programming language
I hope you are aware of the word Syntax. If not so, look, Syntax is a structure or way of writing particular code in a particular programming language. So for more confirmation and knowledge, you can check our detailed post on its Syntax in C#, C++, JAVA, and Python. Now here below is the Syntax of a Variable okay:
[varaible data type] + [varaible name] = [value of variable]
I also make visual representation of a variable hope you like it.
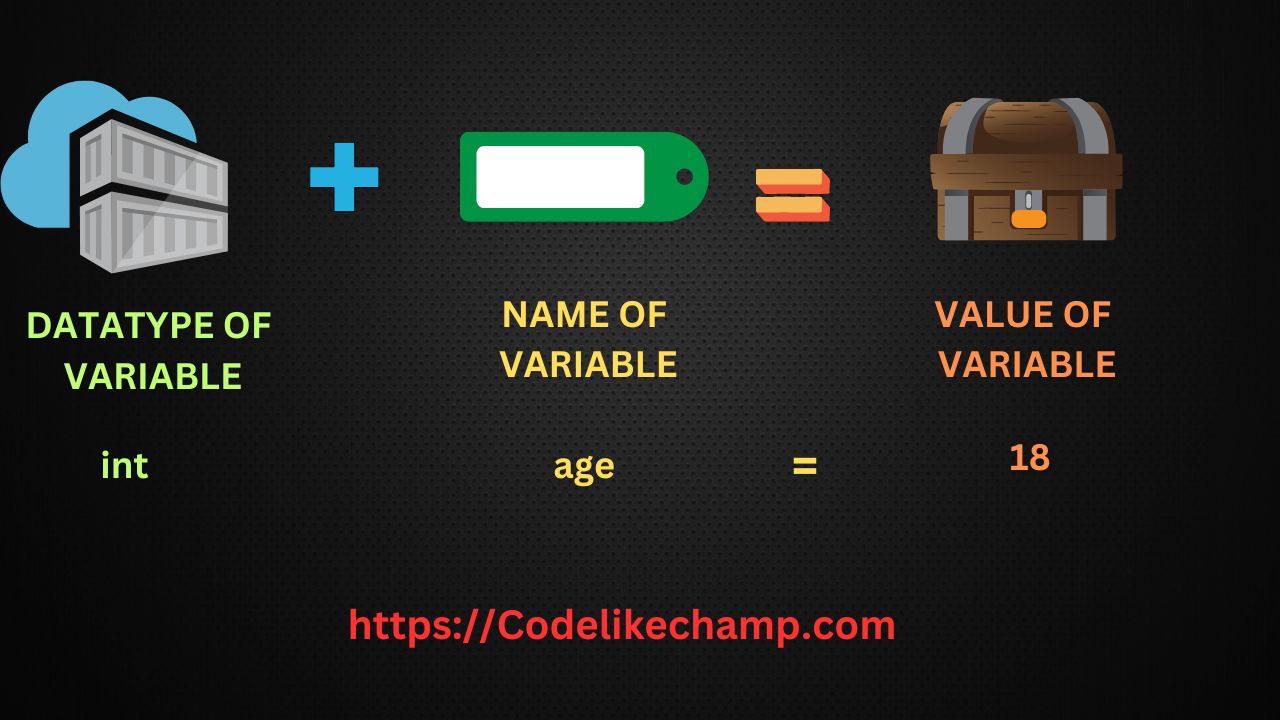
For example : making integers variable age and assigning it value 18 okay using JAVA syntax.
int age =18;
C# Variable in C# Programming language
Now see how ca we declare a variable in C# it is same like as in C++ & JAVA . First we have to create or choose type of variable or you can say datatype of a variable then it’s name and it’s value.
Syntax of C# Variable in C# Programming language
[varaible data type] + [varaible name] = [value of variable]
I also make visual representation of a variable hope you like it.
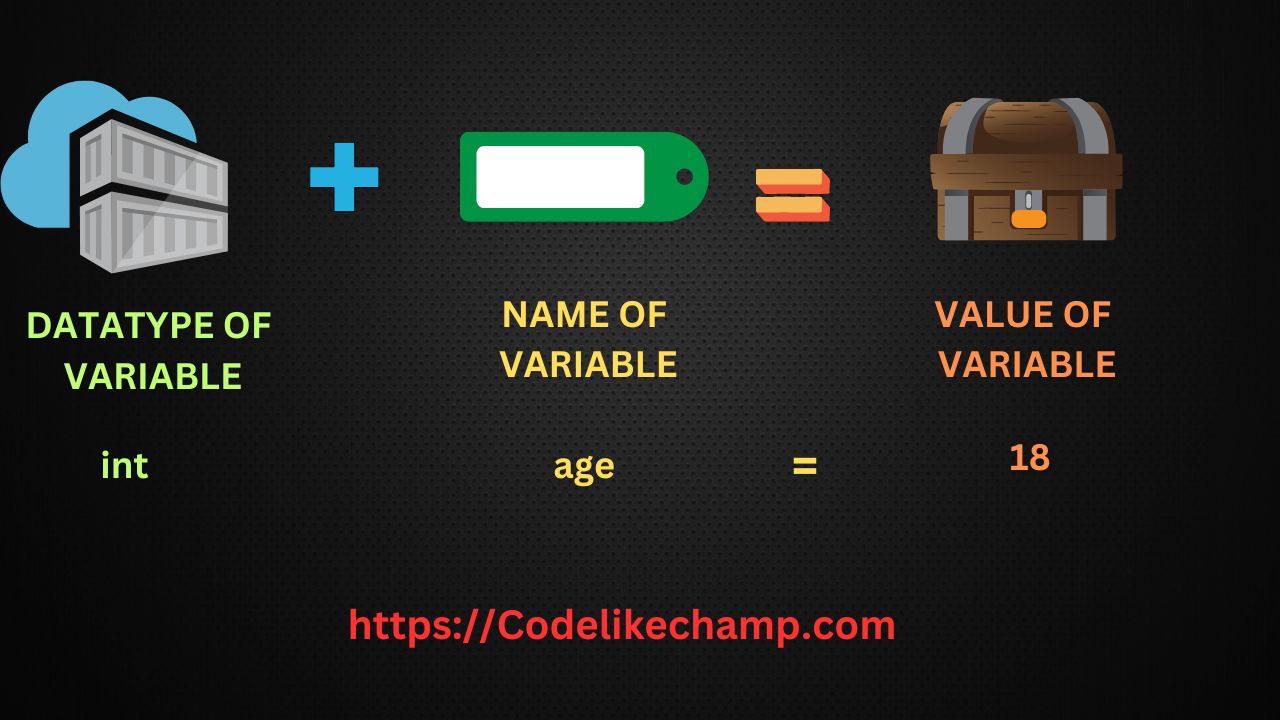
For example : making integers variable age and assigning it value 18 okay using C# syntax.
int age =18;
Other Example:
using System; namespace codelikechamp { class Program { static void Main(string[] args) { int Num; Num = 1000; Console.WriteLine(Num); } } }
Output:
1000
How to create many or multiple C# Variable
We can declare more than one variable of the same type, there are two ways see below:
First way:
using System; namespace codelikechamp { class Program { static void Main(string[] args) { int x = 10; int y = 20; int z = 30; Console.WriteLine(x + y + z); } } }
Output:
60
Second way(it is recommended):
using System; namespace codelikechamp { class Program { static void Main(string[] args) { int x = 10, y = 20, z = 30; Console.WriteLine(x + y + z); } } }
Output:
60
How to assign one value to multiple C# Variable
We can also assign the same value to multiple variables in one line so this reduce our line of code:
First way:
using System; namespace codelikechamp { class Program { static void Main(string[] args) { int x, y, z; x = 10; y = 10; z = 10; Console.WriteLine(x + y + z); } } }
Output:
30
Second way(it is recommended):
using System; namespace codelikechamp { class Program { static void Main(string[] args) { int x, y, z; x = y = z = 10; Console.WriteLine(x + y + z); } } }
Output:
30
Identifiers in C# Variable
All C# variables can be of any datatype and must be identified with unique names. These impressive names are called identifiers. They can be short names (like a and b) or more descriptive names (age, sum, total volume).
Note: It is recommended to avoid short names; instead of this, use descriptive names/variable names to create understandable and maintainable code:
using System; namespace codelikechamp { class Program { static void Main(string[] args) { // Good int secondsPerminute = 60; // OK, but not so easy to understand what s actually is int s = 60; Console.WriteLine(secondsPerminute); Console.WriteLine(s); } } }
Output:
60 60
Rules for naming C# Variable:
The general rules for naming a variables in C# are:
1-Names can contain letters(a,b,c etc), digits(1,2,3), underscores(_), and dollar signs($ etc)
2-Names must begin with a letter.
3-Names should start with a lowercase letter and it cannot contain whitespace
4-Names can also begin with $ and _ (but we will not use it in this blog)
5-Names are case sensitive (“codelikechamp” and “codelikeChamp” are different variables)
6-Reserved words (like C# keywords, such as int or double) cannot be used as names
Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin