Introduction Circular Linked List
In the realm of computer science and software engineering, data structures form the foundation of efficient data organization and manipulation. Among the plethora of data structures, the circular linked list stands as a unique and versatile tool. In this comprehensive article, we delve into the intricacies of circular linked lists, examining their definition, utility, characteristics, types, merits, and demerits.
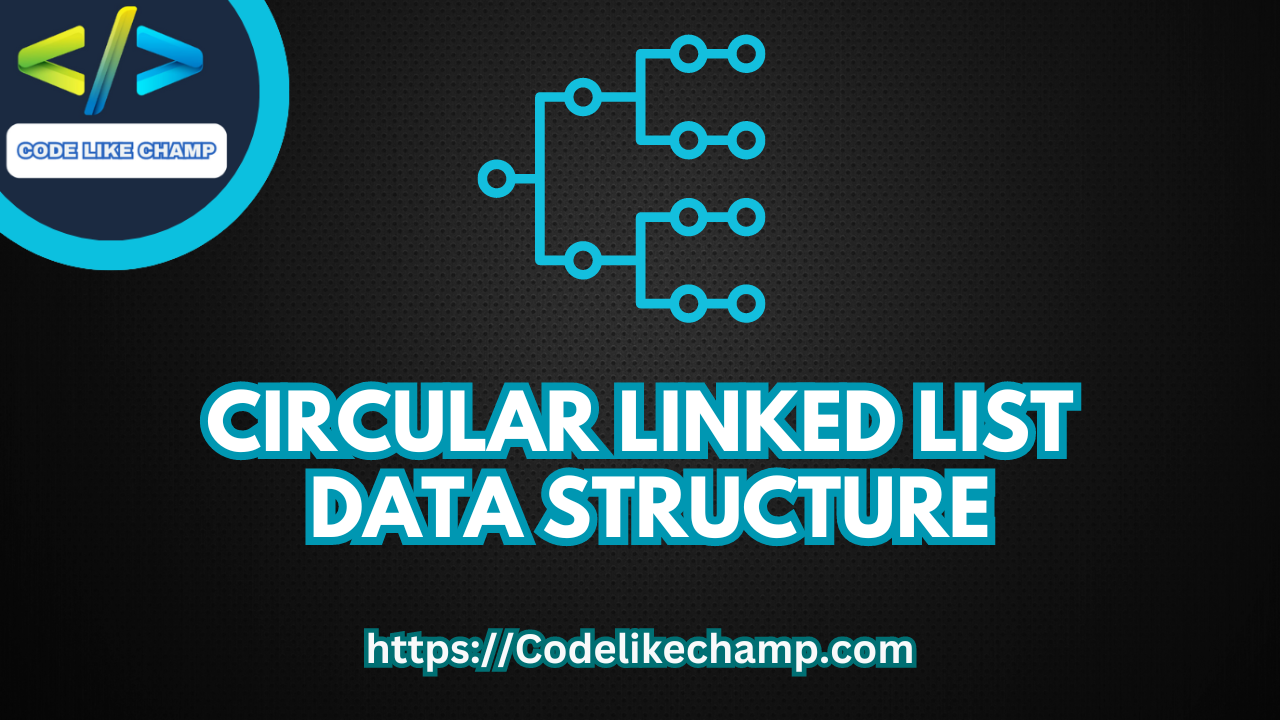
What is Circular Linked Lists
A circular linked list is a variation of the linked list data structure in which the last node points back to the first node, forming a circular structure. Unlike traditional linked lists, where the last node points to null, circular linked lists offer continuous traversal, enabling efficient circular iterations through the list.
Why We Use Circular Linked Lists in Programming
Circular linked lists are used in programming for several reasons:
- Continuous Traversal: Circular linked lists facilitate continuous traversal through the list, allowing for efficient iteration without the need to check for null pointers.
- Circular Buffering: Circular linked lists are employed in implementing circular buffers or ring buffers, enabling efficient data storage and retrieval in applications such as audio processing and networking.
- Resource Management: Circular linked lists are utilized in managing system resources, such as memory allocation and deallocation, by recycling unused resources in a circular manner.
- Algorithmic Solutions: Circular linked lists offer elegant solutions to various algorithmic problems, such as Josephus problem and circular permutation, by leveraging their circular structure.

Characteristics of Circular Linked Lists
- Circular Structure: Each node in a circular linked list contains a pointer/reference to the next node, with the last node pointing back to the first node, forming a circular structure.
- Continuous Traversal: Circular linked lists support continuous traversal through the list, allowing for efficient circular iterations without encountering null pointers.
- Dynamic Size: Circular linked lists can dynamically grow or shrink based on the number of elements added or removed, facilitating efficient memory allocation.
- Loop Detection: Circular linked lists require special handling to detect loops or cycles during traversal, preventing infinite loops and ensuring termination.
Types of Circular Linked Lists
- Singly Linked Circular Lists: In a singly linked circular list, each node contains a single pointer/reference to the next node, forming a unidirectional circular structure.
- Doubly Linked Circular Lists: In a doubly linked circular list, each node contains two pointers/references – one pointing to the previous node and the other pointing to the next node, forming a bidirectional circular structure.
Advantages of Circular Linked Lists
- Continuous Traversal: Circular linkeds lists facilitate continuous traversal through the list, enabling efficient circular iterations without the need for null pointer checks.
- Circular Buffering: Circular linked lists are suitable for implementing circular buffers or ring buffers, providing efficient storage and retrieval of data in cyclic fashion.
- Space Efficiency: Circular linked lists utilize memory efficiently by dynamically allocating memory for each node as needed, minimizing memory wastage.
- Algorithmic Solutions: Circular linked lists offer elegant solutions to various algorithmic problems, such as Josephus problem and circular permutation, by leveraging their circular structure.
Dis-Advantages of Circular Linked Lists
- Loop Detection: Circular linked lists require special handling to detect loops or cycles during traversal, adding complexity to algorithms and potentially impacting performance.
- Increased Memory Overhead: Circular linked lists may incur increased memory overhead due to the maintenance of additional pointers/references for circular linkage, potentially increasing memory usage.
- Complex Implementation: Implementing and managing circular linked lists may require additional considerations, such as loop detection and termination conditions, increasing implementation complexity.
- Limited Applications: Circular linked lists may not be suitable for applications requiring linear traversal or random access to elements, as circular traversal may not preserve the order of elements.
Code Examples of Circular Linked Lists
Below are code examples demonstrating circular linked list usage in various programming languages:
Circular Linked Lists in C#
using System; public class Node { public int data; public Node next; public Node(int d) { data = d; next = null; } } public class CircularLinkedList { public Node head; // Method to print the circular linked list public void PrintList() { if (head == null) return; Node temp = head; do { Console.Write(temp.data + " "); temp = temp.next; } while (temp != head); } } class Program { static void Main(string[] args) { CircularLinkedList cll = new CircularLinkedList(); cll.head = new Node(1); Node second = new Node(2); Node third = new Node(3); cll.head.next = second; second.next = third; third.next = cll.head; // Making it circular cll.PrintList(); } }
Output
1 2 3
Circular Linked Lists in C
#include <stdio.h> #include <stdlib.h> struct Node { int data; struct Node* next; }; void PrintList(struct Node* head) { if (head == NULL) return; struct Node* temp = head; do { printf("%d ", temp->data); temp = temp->next; } while (temp != head); } int main() { struct Node* head = NULL; struct Node* second = NULL; struct Node* third = NULL; head = (struct Node*)malloc(sizeof(struct Node)); second = (struct Node*)malloc(sizeof(struct Node)); third = (struct Node*)malloc(sizeof(struct Node)); head->data = 1; head->next = second; second->data = 2; second->next = third; third->data = 3; third->next = head; // Making it circular PrintList(head); return 0; }
Output
1 2 3
Circular Linked Lists in C++
#include <iostream> class Node { public: int data; Node* next; Node(int d) : data(d), next(nullptr) {} }; class CircularLinkedList { public: Node* head; // Method to print the circular linked list void PrintList() { if (head == nullptr) return; Node* temp = head; do { std::cout << temp->data << " "; temp = temp->next; } while (temp != head); } }; int main() { CircularLinkedList cll; cll.head = new Node(1); Node* second = new Node(2); Node* third = new Node(3); cll.head->next = second; second->next = third; third->next = cll.head; // Making it circular cll.PrintList(); return 0; }
Output
1 2 3
Circular Linked Lists in Python
class Node: def __init__(self, data): self.data = data self.next = None class CircularLinkedList: def __init__(self): self.head = None # Method to print the circular linked list def PrintList(self): if self.head is None: return temp = self.head while True: print(temp.data, end=" ") temp = temp.next if temp == self.head: break cll = CircularLinkedList() cll.head = Node(1) second = Node(2) third = Node(3) cll.head.next = second second.next = third third.next = cll.head # Making it circular cll.PrintList()
Output
1 2 3
Circular Linked Lists in PHP
<?php class Node { public $data; public $next; public function __construct($d) { $this->data = $d; $this->next = null; } } class CircularLinkedList { public $head; // Method to print the circular linked list public function PrintList() { if ($this->head === null) return; $temp = $this->head; do { echo $temp->data . " "; $temp = $temp->next; } while ($temp !== $this->head); } } $cll = new CircularLinkedList(); $cll->head = new Node(1); $second = new Node(2); $third = new Node(3); $cll->head->next = $second; $second->next = $third; $third->next = $cll->head; // Making it circular $cll->PrintList(); ?>
Output
1 2 3
Circular Linked Lists in JAVA
class Node { int data; Node next; Node(int d) { data = d; next = null; } } class CircularLinkedList { Node head; // Method to print the circular linked list void PrintList() { if (head == null) return; Node temp = head; do { System.out.print(temp.data + " "); temp = temp.next; } while (temp != head); } } public class Main { public static void main(String[] args) { CircularLinkedList cll = new CircularLinkedList(); cll.head = new Node(1); Node second = new Node(2); Node third = new Node(3); cll.head.next = second; second.next = third; third.next = cll.head; // Making it circular cll.PrintList(); } }
Output
1 2 3
Circular Linked Lists in JavaScript
class Node { constructor(data) { this.data = data; this.next = null; } } class CircularLinkedList { constructor() { this.head = null; } // Method to print the circular linked list PrintList() { if (this.head === null) return; let temp = this.head; do { console.log(temp.data + " "); temp = temp.next; } while (temp !== this.head); } } let cll = new CircularLinkedList(); cll.head = new Node(1); let second = new Node(2); let third = new Node(3); cll.head.next = second; second.next = third; third.next = cll.head; // Making it circular cll.PrintList();
Output
1 2 3
Conclusion
In conclusion, circular linked lists represent a versatile and efficient data structure, offering continuous traversal, space efficiency, and elegant solutions to various algorithmic problems. Despite their complexities and potential overhead, circular linked lists find widespread applications in implementing circular buffers, managing system resources, and solving algorithmic challenges. By mastering the concepts and applications of circular linked lists, programmers can enhance their problem-solving skills and develop robust, efficient algorithms, contributing to the advancement of technology and innovation in the digital age.
For more visit my website Codelikechamp.com