Introduction to Linked List
In the realm of computer science and programming, understanding data structures is paramount. Among these structures, linked list stand as fundamental pillars, offering versatile solutions to various computational challenges. In this comprehensive guide, we explore the intricacies of linked lists, shedding light on their definition, usage, characteristics, types, merits, and demerits.
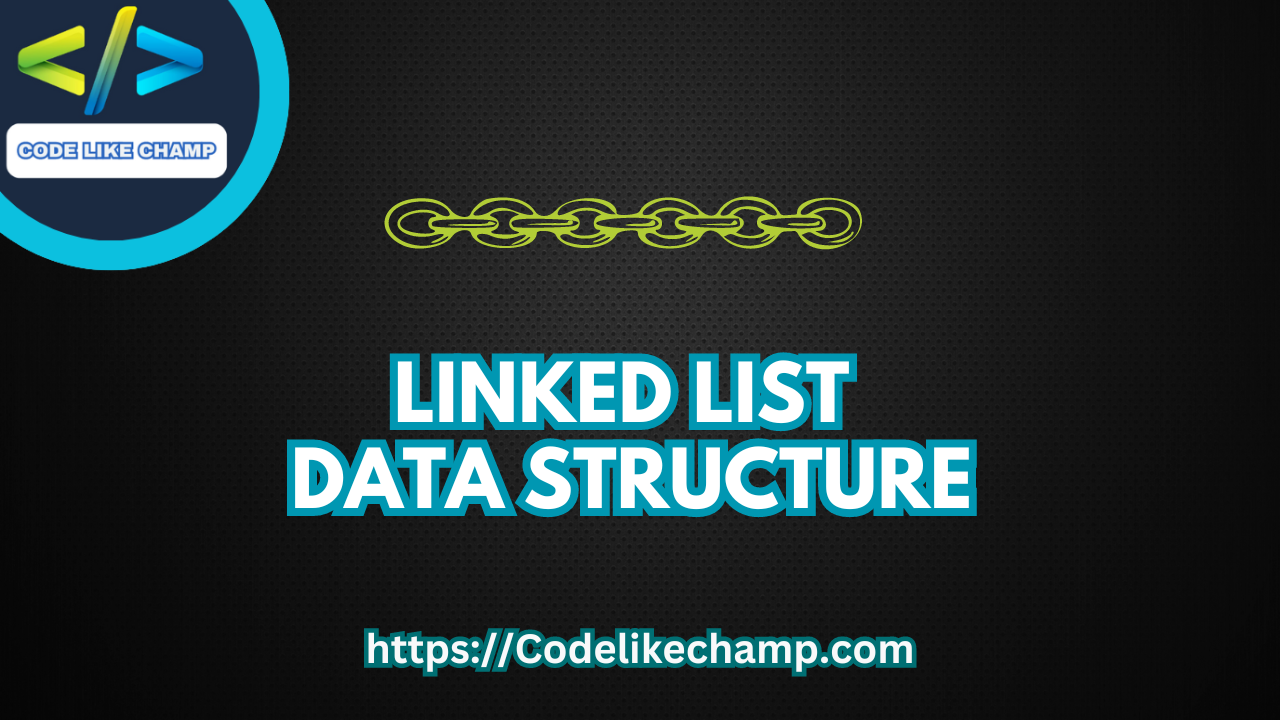
What is a Linked Lists
A linked list is a linear data structure comprising a sequence of elements, known as nodes, where each node contains a value and a reference (or pointer) to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation, allowing for dynamic memory allocation and efficient insertion and deletion operations.
Why We Use Linked Lists in Programming
Linked lists are employed in programming for several reasons:
- Dynamic Memory Management: Linked lists facilitate dynamic memory allocation, enabling efficient memory utilization and management.
- Flexibility in Insertions and Deletions: Linked lists support efficient insertion and deletion operations anywhere within the list, without the need for shifting elements.
- Versatile Applications: Linked lists find applications in various scenarios, including implementing stacks, queues, and adjacency lists for graphs.
- Scalability: Linked lists can scale dynamically to accommodate varying data sizes, making them suitable for dynamic environments.
Characteristics of Linked Lists
- Dynamic Size: Linked lists can grow or shrink dynamically based on the number of elements added or removed.
- Node Structure: Each node in a linked list consists of two parts: data and a reference/pointer to the next node.
- Sequential Access: Linked lists support sequential access, starting from the head (first node) and traversing through subsequent nodes.
- No Contiguous Memory Allocation: Unlike arrays, linked lists do not require contiguous memory allocation, allowing for efficient memory usage.
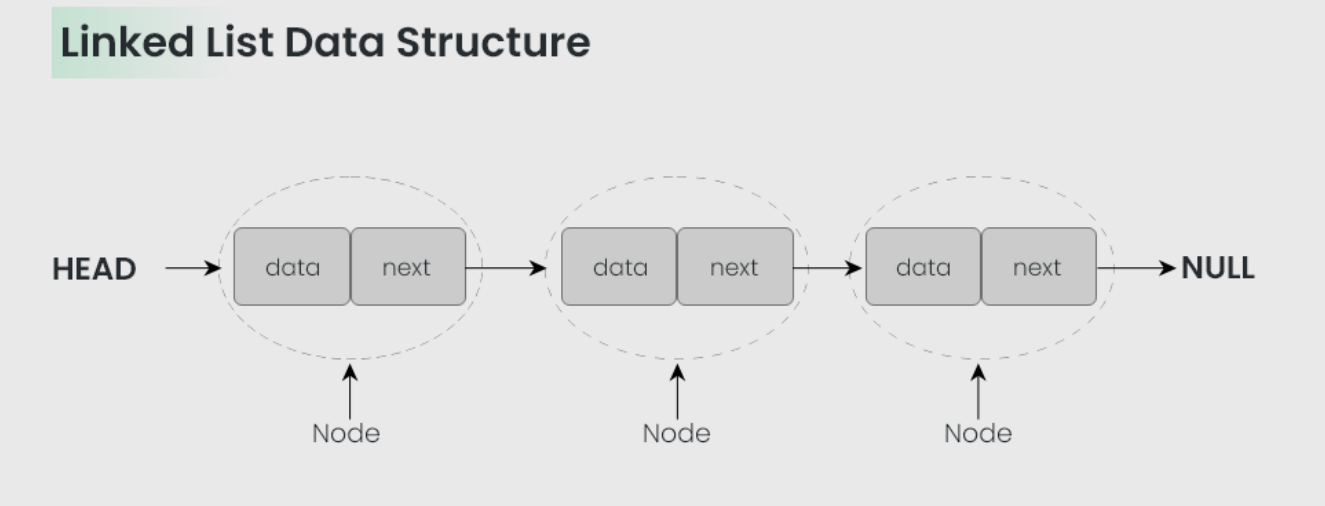
Types of Linked Lists
- Singly Linked List: In a singly linked list, each node contains data and a pointer to the next node in the sequence.
- Doubly Linked List: A doubly linked list extends the concept of a singly linked list by each node containing an additional pointer to the previous node.
- Circular Linked Lists: In a circular linked list, the last node points back to the first node, forming a circular structure.
- Sorted Linked Lists: Sorted linked lists maintain elements in sorted order, facilitating efficient searching and insertion.
Advantages of Linked Lists data structure
- Dynamic Memory Allocation: Linked lists facilitate dynamic memory allocation, accommodating varying data sizes.
- Efficient Insertions and Deletions: Linked lists support efficient insertion and deletion operations, especially in large datasets.
- Flexibility: Linked lists offer flexibility in managing data structures, allowing for dynamic rearrangement of elements.
- Versatility: Linked lists serve as building blocks for implementing other data structures and algorithms, such as stacks and queues.
Dis-Advantages of Linked Lists data structure
- Lack of Random Access: Linked lists do not support direct access to elements by index, necessitating sequential traversal for access.
- Overhead of Pointers: Each node in a linked list requires additional memory overhead for storing pointers, potentially increasing memory usage.
- Increased Complexity: Manipulating linked lists may require additional considerations, such as handling null pointers and edge cases.
- Inefficient Memory Usage: Linked lists may suffer from inefficient memory usage due to memory fragmentation and pointer overhead.
Code Examples of Linked Lists data structure
Below are code examples demonstrating linked list usage in various programming languages:
Linked Lists C#
using System; class Node { public int data; public Node next; public Node(int d) { data = d; next = null; } } class LinkedList { Node head; public void PrintList() { Node n = head; while (n != null) { Console.Write(n.data + " "); n = n.next; } Console.WriteLine(); } } class Program { static void Main(string[] args) { LinkedList list = new LinkedList(); list.head = new Node(1); list.head.next = new Node(2); list.head.next.next = new Node(3); list.PrintList(); } }
Output
1 2 3
Linked Lists in C
#include <stdio.h> #include <stdlib.h> struct Node { int data; struct Node* next; }; void printList(struct Node* n) { while (n != NULL) { printf("%d ", n->data); n = n->next; } printf("\n"); } int main() { struct Node* head = NULL; struct Node* second = NULL; struct Node* third = NULL; head = (struct Node*)malloc(sizeof(struct Node)); second = (struct Node*)malloc(sizeof(struct Node)); third = (struct Node*)malloc(sizeof(struct Node)); head->data = 1; head->next = second; second->data = 2; second->next = third; third->data = 3; third->next = NULL; printList(head); return 0; }
Output
1 2 3
Linked Lists C++
#include <iostream> class Node { public: int data; Node* next; Node(int d) : data(d), next(nullptr) {} }; class LinkedList { public: Node* head; LinkedList() : head(nullptr) {} void printList() { Node* n = head; while (n != nullptr) { std::cout << n->data << " "; n = n->next; } std::cout << std::endl; } }; int main() { LinkedList list; list.head = new Node(1); list.head->next = new Node(2); list.head->next->next = new Node(3); list.printList(); return 0; }
Output
1 2 3
Linked Lists Python
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def printList(self): n = self.head while n is not None: print(n.data, end=" ") n = n.next print() list = LinkedList() list.head = Node(1) list.head.next = Node(2) list.head.next.next = Node(3) list.printList()
Output
1 2 3
Linked Lists PHP
<?php class Node { public $data; public $next; function __construct($d) { $this->data = $d; $this->next = null; } } class LinkedList { public $head; function printList() { $n = $this->head; while ($n != null) { echo $n->data . " "; $n = $n->next; } echo "\n"; } } $list = new LinkedList(); $list->head = new Node(1); $list->head->next = new Node(2); $list->head->next->next = new Node(3); $list->printList(); ?>
Output
1 2 3
Linked Lists JAVA
class Node { int data; Node next; Node(int d) { data = d; next = null; } } class LinkedList { Node head; void printList() { Node n = head; while (n != null) { System.out.print(n.data + " "); n = n.next; } System.out.println(); } } public class Main { public static void main(String[] args) { LinkedList list = new LinkedList(); list.head = new Node(1); list.head.next = new Node(2); list.head.next.next = new Node(3); list.printList(); } }
Output
1 2 3
Linked Lists JavaScript
class Node { constructor(data) { this.data = data; this.next = null; } } class LinkedList { constructor() { this.head = null; } printList() { let n = this.head; while (n !== null) { process.stdout.write(n.data + " "); n = n.next; } console.log(); } } let list = new LinkedList(); list.head = new Node(1); list.head.next = new Node(2); list.head.next.next = new Node(3); list.printList();
Output
1 2 3
Conclusion to Linked lists
Linked lists serve as indispensable tools in the realm of data structures and programming, offering dynamic memory allocation, efficient insertion and deletion operations, and versatile applications. Despite their limitations such as lack of random access and overhead of pointers, linked lists remain invaluable in various domains, ranging from implementing basic data structures to addressing complex computational challenges. By grasping the nuances of linked lists, programmers can harness their power to develop efficient algorithms and robust applications, contributing to the advancement of technology and innovation.
For more visit my website Codelikechamp.com