Introduction: In the realm of computer programming and data management, arrays stand as one of the foundational data structures. Array provide a structured and efficient way to store and manipulate collections of elements, forming the backbone of many algorithms and applications. In this comprehensive guide, we delve into the intricacies of arrays, exploring their purpose, characteristics, types, merits, and demerits.
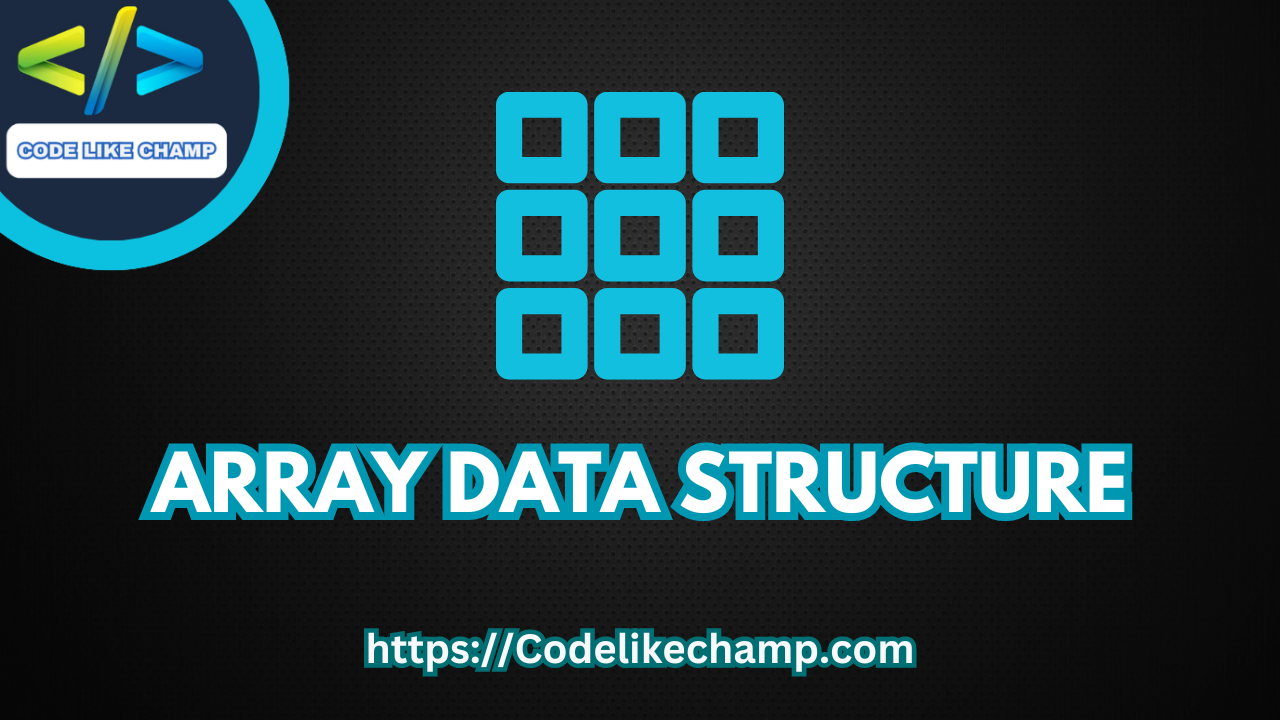
What is an Array?
An array is a contiguous collection of elements, each identified by an index or key. These elements are stored in a linear fashion, occupying adjacent memory locations. Arrays offer a means to organize and access data in a systematic manner, facilitating efficient retrieval and manipulation.
Why We Use it in Programming
Arrays play a pivotal role in programming for several reasons:
- Efficient Storage: Arrays enable efficient storage of elements in a contiguous block of memory, allowing for rapid access and manipulation.
- Indexed Access: Elements within an array are accessed using their index, providing direct and fast retrieval.
- Iteration: Arrays facilitate easy iteration over elements, making it simple to perform operations on each element sequentially.
- Versatility: Arrays can store elements of the same data type or heterogeneous elements, offering flexibility in data representation.
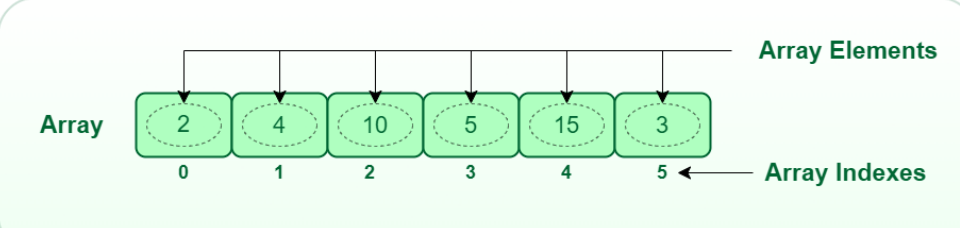
Characteristics of Array
- Fixed Size: Arrays have a fixed size determined at the time of declaration, which cannot be dynamically changed during runtime.
- Homogeneous: Arrays typically store elements of the same data type, ensuring uniformity in data representation.
- Random Access: Elements in an array can be accessed directly using their index, enabling constant-time access.
- Contiguous Memory Allocation: Array elements are stored in consecutive memory locations, facilitating efficient memory access and traversal.
Types of Array
- One-dimensional Array: A single-dimensional array stores elements in a linear sequence, accessed using a single index.
- Multi-dimensional Array: Multi-dimensional arrays store elements in multiple dimensions, such as rows and columns in a matrix.
Advantages of array data structure
- Efficient Access: Arrays allow for fast and direct access to elements using their index.
- Memory Efficiency: Arrays utilize contiguous memory allocation, minimizing memory overhead.
- Versatility: Arrays support various operations, including insertion, deletion, and searching.
- Simplified Iteration: Arrays simplify the process of iterating over elements, facilitating algorithmic implementations.
Dis-Advantages of array data structure
- Fixed Size: The fixed size of arrays can lead to memory wastage if not utilized fully or constraints if additional space is required.
- Lack of Dynamic Resize: Arrays cannot be dynamically resized, requiring preallocation of memory based on the maximum expected size.
- Homogeneity Requirement: Arrays typically store elements of the same data type, limiting their flexibility in handling heterogeneous data.
- Inefficient Insertion/Deletion: Insertion or deletion operations in arrays may require shifting elements, leading to inefficiency, especially for large arrays.
Code Examples of array
Below are code examples demonstrating array usage in various programming languages:
Arrays in C#
using System; class Program { static void Main(string[] args) { int[] arr = {1, 2, 3, 4, 5}; foreach (int num in arr) { Console.Write(num + " "); } } }
Output
1 2 3 4 5
Arrays in C
#include <stdio.h> int main() { int arr[] = {1, 2, 3, 4, 5}; int n = sizeof(arr) / sizeof(arr[0]); for (int i = 0; i < n; i++) { printf("%d ", arr[i]); } return 0; }
Output
1 2 3 4 5
Arrays in C++
#include <iostream> int main() { int arr[] = {1, 2, 3, 4, 5}; int n = sizeof(arr) / sizeof(arr[0]); for (int i = 0; i < n; i++) { std::cout << arr[i] << " "; } return 0; }
Output
1 2 3 4 5
Arrays in Python
arr = [1, 2, 3, 4, 5] for num in arr: print(num, end=" ")
Output
1 2 3 4 5
Arrays in PHP
<?php $arr = array(1, 2, 3, 4, 5); foreach ($arr as $num) { echo $num . " "; } ?>
Output
1 2 3 4 5
Arrays in JAVA
public class Main { public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5}; for (int num : arr) { System.out.print(num + " "); } } }
Output
1 2 3 4 5
Array in JavaScript
let arr = [1, 2, 3, 4, 5]; arr.forEach(num => console.log(num));
Output
1 2 3 4 5
Conclusion
Arrays represent a fundamental data structure in computer science and programming, offering efficient storage, fast access, and versatile manipulation of data. While arrays boast several merits such as efficient access and memory usage, they also exhibit limitations, including fixed size and lack of dynamic resizing. Despite their shortcomings, arrays remain indispensable in various domains, serving as the building blocks for many algorithms and applications. By understanding the characteristics, types, merits, and demerits of arrays, programmers can leverage their power effectively to solve a myriad of computational problems.
For more visit my website Codelikechamp.com