Introduction to Deque (Double ended queue)
In the realm of computer science and programming, efficient data handling is paramount. One such crucial data structure that aids in this endeavor is the Deque, short for Double Ended Queue. This article will delve into what a Deque is, its importance in programming, its characteristics, types, advantages, disadvantages, and provide code snippets in various programming languages to illustrate its usage.

What is Deques (Double Ended Queue)
A Deque, pronounced as “deck,” is a linear data structure that allows insertion and deletion of elements from both the front and the rear ends. Essentially, it combines the features of both stacks and queues, enabling versatile data manipulation operations.
Why We Use Deques in Programming
Deques find extensive utility in programming due to their flexibility and efficiency in managing data. They offer constant time complexity for insertion and deletion operations at both ends, making them ideal for scenarios requiring dynamic data handling, such as implementing algorithms like breadth-first search, reversing elements, etc.
Characteristics of Deques (Double ended queue)
- Allows insertion and deletion from both ends
- Maintains the order of elements
- Offers constant time complexity for basic operations
- Supports dynamic resizing for efficient memory utilization
Types of Deques (Double ended queue)
- Array-based Deque: Implemented using arrays, allowing efficient random access to elements.
- Linked-list based Deque: Implemented using linked lists, offering flexibility in memory allocation and dynamic resizing.
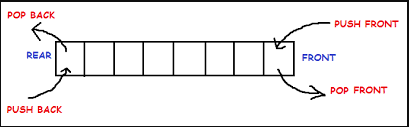
Advantages of Deques
- Efficient insertion and deletion operations at both ends
- Versatile data handling capabilities
- Supports various programming paradigms
- Suitable for implementing algorithms requiring dynamic data manipulation
Disadvantages of Deques
- May incur overhead due to dynamic resizing
- Array-based implementation may suffer from memory fragmentation
- Linked-list based implementation may have higher memory overhead due to node pointers
Code Snippets of Deques (Double ended queue)
Deques in C#
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { Deque<int> deque = new Deque<int>(); deque.AddFirst(1); deque.AddLast(2); deque.AddFirst(3); foreach (var item in deque) { Console.WriteLine(item); } } }
Output
3 1 2
Deques in C
#include <stdio.h> #include <stdlib.h> struct Node { int data; struct Node* next; struct Node* prev; }; typedef struct Node Node; Node* createNode(int data) { Node* newNode = (Node*)malloc(sizeof(Node)); newNode->data = data; newNode->next = NULL; newNode->prev = NULL; return newNode; } void main() { Node* front = createNode(1); Node* rear = createNode(2); front->next = rear; rear->prev = front; printf("Front: %d\n", front->data); printf("Rear: %d\n", rear->data); }
Output
Front: 1 Rear: 2
Deques in C++
#include <iostream> #include <deque> int main() { std::deque<int> deque; deque.push_front(1); deque.push_back(2); deque.push_front(3); for (auto it = deque.begin(); it != deque.end(); ++it) { std::cout << *it << std::endl; } return 0; }
Output
3 1 2
Deque in Python
from collections import deque deque = deque() deque.appendleft(1) deque.append(2) deque.appendleft(3) for item in deque: print(item)
Output
3 1 2
Deques in PHP
<?php $deque = new SplDoublyLinkedList(); $deque->push(1); $deque->unshift(2); $deque->push(3); $deque->rewind(); while ($deque->valid()) { echo $deque->current() . "\n"; $deque->next(); } ?>
Output
2 1 3
Deques in JAVA
import java.util.Deque; import java.util.ArrayDeque; public class Main { public static void main(String[] args) { Deque<Integer> deque = new ArrayDeque<>(); deque.addFirst(1); deque.addLast(2); deque.addFirst(3); for (int item : deque) { System.out.println(item); } } }
Output
3 1 2
Deque in JavaScript
let deque = []; deque.unshift(1); deque.push(2); deque.unshift(3); for (let item of deque) { console.log(item); }
Output
3 1 2
Conclusion
In conclusion, Deques (Double Ended Queue) stands as a versatile data structure offering efficient insertion and deletion operations from both ends. Its characteristics, types, advantages, and disadvantages highlight its significance in programming, where dynamic data manipulation is paramount. By understanding and leveraging Deque in various programming languages, developers can enhance their algorithmic efficiency and optimize data handling in their applications.
For more visit my website Codelikechamp.com