Introduction to Loop through List C#
In the realm of programming, efficient data manipulation is key, and one of the most fundamental tasks is traversing through a collection of items. In C#, a popular and versatile programming language, the process of looping through a list is an essential skill every developer should master. Whether you’re a novice or an experienced coder, this article will guide to go through how to Loop through List C#, providing insights, code snippets, and practical examples. I hope you like my this article and if you face any problem in it so please contact me without any hesitation or ask in comment section.
Table of Contents
- Introduction
- The Significance of Efficient Data Manipulation
- Mastering List Iteration in C#: An Essential Skill
- Understanding Lists and Their Importance
- Lists: Your Toolbox for Efficient Data Management
- Dynamic Sizing and Versatility: Advantages of Lists
- Simplifying Data Manipulation with List Methods
- The For Loop Method
- Exploring the Traditional for Loop
- Fine-Grained Control and Index Access
- Sample Code: Iterating with the for Loop
- The Foreach Loop Technique
- Embracing the Graceful foreach Loop
- A New Level of Readability and Index Management
- Practical Example: Walking Through Elements
- Leveraging LINQ for List Iteration
- Introducing the Power of Language Integrated Query (LINQ)
- Complex Queries and Transformations Made Easy
- Putting LINQ into Action: Filtering and Iteration
- The While Loop Approach
- The Flexible Approach of the while Loop
- Conditional Traversal: When while Loop Shines
- Example: Navigating a List with while
- Best Practices for Efficient List Iteration
- The Preferred Approach: Benefits of the foreach Loop
- Elevating List Manipulation with LINQ
- Choosing the Right List Type: List<T> vs. LinkedList<T>
- When Indices Rule: Making the Most of the for Loop
- Conclusion
- The Crucial Skill of Mastering List Iteration
- Unleashing the Power of Different Looping Techniques
- Elevating Code Readability and Performance
- References
- Microsoft Docs – List<T> Class
- Microsoft Docs – foreach, in
- Microsoft Docs – LINQ Query Operators
- C# Corner – Different Ways to Iterate Over a List in C#
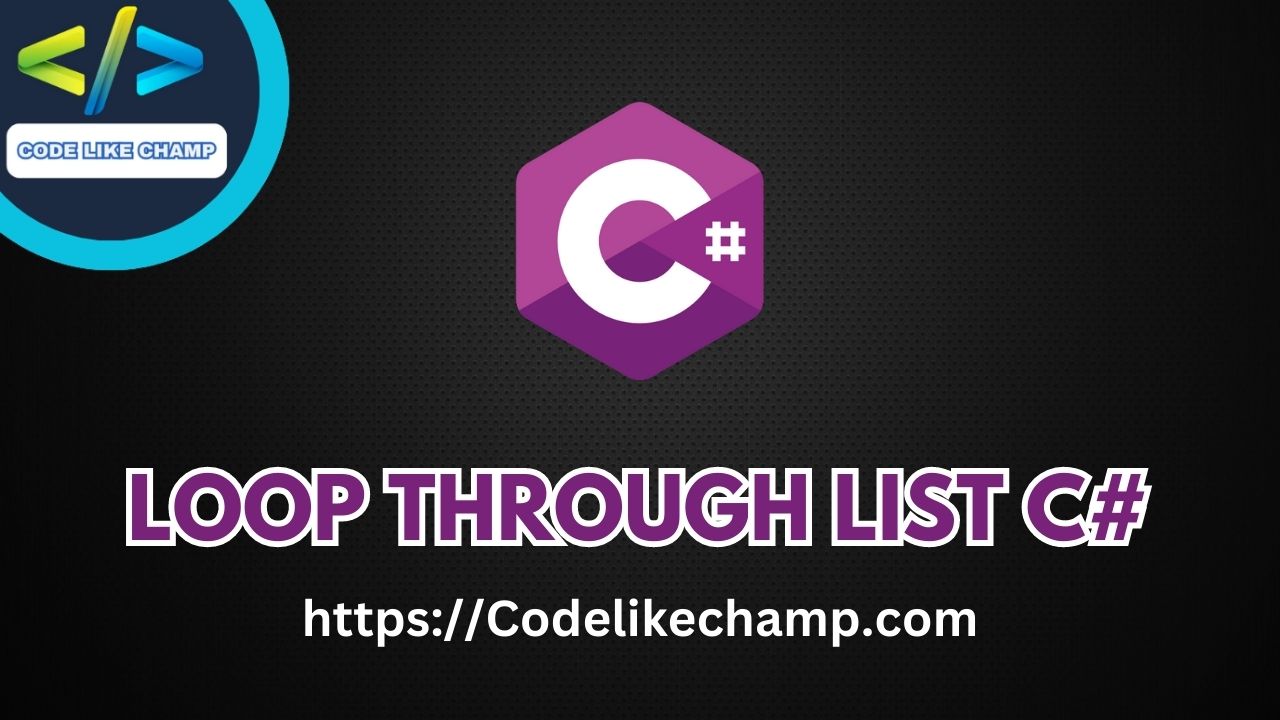
Understanding Lists and Their Importance
Before we start learning about looping through lists in C#, let’s understand why lists are important in programming. Lists are like containers that let you store and handle bunches of things in a smart way. They’re great because they can change in size as you need, they’re really flexible, and they come with lots of tools that make working with data easier. So, they’re basically super handy when you want to work with a bunch of items together.
The For Loop Method to Loop through List C#
The traditional for loop is a staple in C# programming for iterating through a list. It provides fine-grained control over the iteration process and is especially useful when you need to access list elements by their index. See below example for more clarification.
List<string> fruits = new List<string>{"apple", "banana", "orange", "grape"}; for (int i = 0; i < fruits.Count; i++) { Console.WriteLine(fruits[i]); }
The Foreach Loop Technique to Loop through List C#
The foreach loop is an elegant and reader-friendly approach to traverse through a list in C#. It automatically takes care of index management and provides a concise syntax for iteration. See below example for more clarification.
List<int> numbers = new List<int>{1, 2, 3, 4, 5}; foreach (int number in numbers) { Console.WriteLine(number); }
LINQ for List Iteration
Language Integrated Query (LINQ) introduces a powerful way to manipulate collections in C#. It allows you to perform complex queries and transformations on lists, making iteration more efficient and expressive. See below example for more clarification.
List<int> scores = new List<int>{85, 92, 78, 95, 88}; var passingScores = scores.Where(score => score >= 80); foreach (var score in passingScores) { Console.WriteLine(score); }
The While Loop Approach to Loop through List C#
The while loop offers a flexible iterative mechanism that allows you to loop through a list based on a specific condition. While less common for list iteration, it can be useful in scenarios requiring conditional traversal. See below example for more clarification.
List<string> daysOfWeek = new List<string>{"Monday", "Tuesday", "Wednesday", "Thursday", "Friday"}; int index = 0; while (index < daysOfWeek.Count) { Console.WriteLine(daysOfWeek[index]); index++; }
Best Practices for Efficient List Iteration
- Prefer foreach: Whenever possible, opt for the foreach loop, as it simplifies code and minimizes potential errors related to index manipulation.
- Use LINQ for Complex Operations: For intricate data manipulations and filtering, leverage LINQ queries to enhance readability and maintainability.
- Consider List Type: Choose the appropriate list type based on your needs. For example, if you require fast random access, use List<T>, but if memory usage is a concern, consider LinkedList<T>.
- Use for Loop for Index-Dependent Operations: When your iteration relies on indices, the traditional for loop is still your best bet.
Conclusion to Loop through List C#
Now in last i want to conclude by saying this that, looping through lists in C# is a fundamental skill that lays the foundation for efficient data manipulation and algorithm implementation. Whether you’re iterating using the classic for loop, the elegant foreach loop, or harnessing the power of LINQ, understanding these techniques is paramount. By choosing the right iteration method for each scenario, you’ll not only improve code readability but also enhance the overall performance of your applications.
References
- Microsoft Docs – List<T> Class
- Microsoft Docs – foreach, in
- Microsoft Docs – LINQ Query Operators
- C# Corner – Different Ways to Iterate Over a List in C#
As you embark on your journey to become a proficient C# developer, mastering the art of looping through lists will undoubtedly serve as a cornerstone of your programming prowess.
Link: https://Codelikechamp.com
Medium Link: Follow me on Medium
Linkedin Link: Follow me on Linkedin