Introduction to Static Array
In the realm of data structures, arrays stand as one of the foundational building blocks. Among them, the static array holds a unique position, offering distinct advantages and limitations. In this article, we delve into the concept of static arrays, exploring their characteristics, types, applications, advantages, and disadvantages.
WANT TO PRO IN DATA STRUCTURE JUST VISIT THIS PAGE IT WILL BE BENEFICIAL FOR YOU –>> DATA STRUCTURE
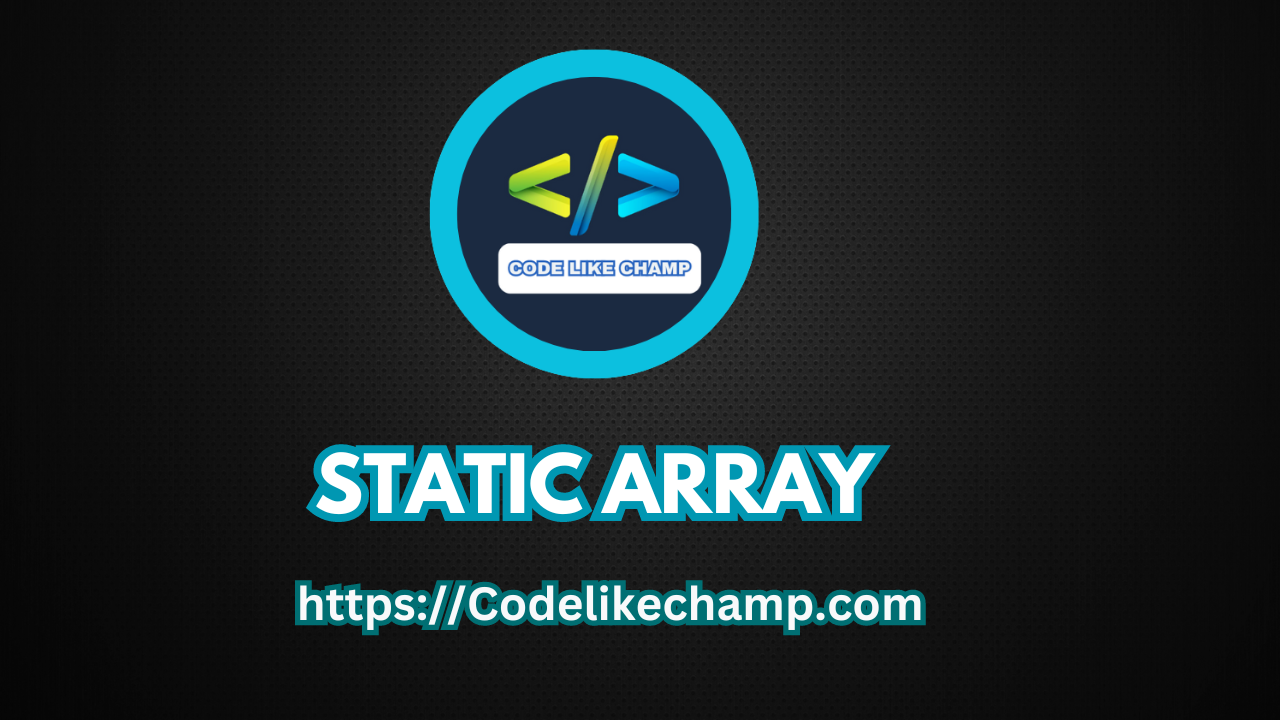
What is a Static Arrays?
A static array, also known as a fixed-size array, is a data structure that stores elements of the same data type in contiguous memory locations. Unlike dynamic arrays, the size of a static array is fixed at compile time and cannot be altered during runtime.
Why We Use Static Arrays in Data Structure
Static arrays find extensive use in situations where the size of the data collection is known beforehand and remains constant throughout the program’s execution. They provide efficient access to elements through constant-time indexing, making them suitable for scenarios requiring fast element retrieval.
Characteristics of Static Arrays:
- Fixed Size: The size of a static array is predetermined and remains unchanged throughout its lifetime.
- Contiguous Memory Allocation: Elements of a static array are stored in adjacent memory locations, enabling efficient memory access.
- Constant-Time Access: Accessing elements in a static array is achieved in constant time, O(1), through direct indexing.
Types of Static Arrays:
While the concept of static arrays remains consistent across programming languages, their implementation may vary. However, there are no distinct types of static arrays; they are simply arrays with a fixed size.
Advantages of Static Arrays:
- Efficiency: Static arrays offer constant-time access to elements, making them efficient for read and write operations.
- Predictability: The fixed size of static arrays provides predictability in memory usage and performance, aiding in resource management.
- Simplicity: Static arrays are straightforward to implement and use, requiring minimal overhead.
Disadvantages of Static Array:
- Fixed Size Limitation: The inability to resize static arrays dynamically limits their flexibility, making them unsuitable for scenarios with varying data sizes.
- Memory Wastage: Static arrays allocate memory for their maximum capacity, even if the actual data size is smaller, leading to potential memory wastage.
- Stack Size Restrictions: In some programming languages, static arrays declared within functions are allocated on the stack, subjecting them to stack size limitations.
Code Examples and Outputs:
Let’s explore implementations of a static array in various programming languages:
Static Arrays in C#
using System; class Program { static void Main(string[] args) { int[] staticArray = new int[5] { 1, 2, 3, 4, 5 }; // Accessing elements Console.WriteLine("Elements of static array:"); foreach (int element in staticArray) { Console.Write(element + " "); } } }
Output
Elements of static array: 1 2 3 4 5
Static Arrays in C
#include <stdio.h> int main() { int staticArray[5] = {1, 2, 3, 4, 5}; // Accessing elements printf("Elements of static array:\n"); for (int i = 0; i < 5; i++) { printf("%d ", staticArray[i]); } return 0; }
Output
Elements of static array: 1 2 3 4 5
Static Arrays in C++
#include <iostream> int main() { int staticArray[5] = {1, 2, 3, 4, 5}; // Accessing elements std::cout << "Elements of static array:" << std::endl; for (int i = 0; i < 5; i++) { std::cout << staticArray[i] << " "; } return 0; }
Output
Elements of static array: 1 2 3 4 5
Static Arrays in Python
staticArray = [1, 2, 3, 4, 5] # Accessing elements print("Elements of static array:") for element in staticArray: print(element, end=" ")
Output
Elements of static array: 1 2 3 4 5
Static Arrays in PHP
<?php $staticArray = array(1, 2, 3, 4, 5); // Accessing elements echo "Elements of static array:\n"; foreach ($staticArray as $element) { echo $element . " "; } ?>
Output
Elements of static array: 1 2 3 4 5
Static Array in JAVA
public class Main { public static void main(String[] args) { int[] staticArray = {1, 2, 3, 4, 5}; // Accessing elements System.out.println("Elements of static array:"); for (int element : staticArray) { System.out.print(element + " "); } } }
Output
Elements of static array: 1 2 3 4 5
Static Array in JavaScript
let staticArray = [1, 2, 3, 4, 5]; // Accessing elements console.log("Elements of static array:"); staticArray.forEach(element => { console.log(element); });
Output
Elements of static array: 1 2 3 4 5
Conclusion
Static arrays play a crucial role in data structure implementations, offering fast and predictable access to elements. While they come with advantages such as efficiency and simplicity, their fixed size limitation and memory wastage pose challenges in certain scenarios. Understanding the characteristics and nuances of static arrays equips programmers to leverage their strengths effectively while mitigating their limitations in software development endeavors.
For more visit my website Codelikechamp.com
I do agree with all the ideas you have introduced on your post They are very convincing and will definitely work Still the posts are very short for newbies May just you please prolong them a little from subsequent time Thank you for the post
I do trust all the ideas youve presented in your post They are really convincing and will definitely work Nonetheless the posts are too short for newbies May just you please lengthen them a bit from next time Thank you for the post