Introduction to Stack
In the realm of computer science and software engineering, the concept of data structures forms the cornerstone of efficient algorithm design and problem-solving. Among the myriad of data structures, the stack stands out as a fundamental and versatile tool. In this comprehensive article, we delve into the intricacies of stacks, exploring their definition, utility, characteristics, types, merits, and demerits.
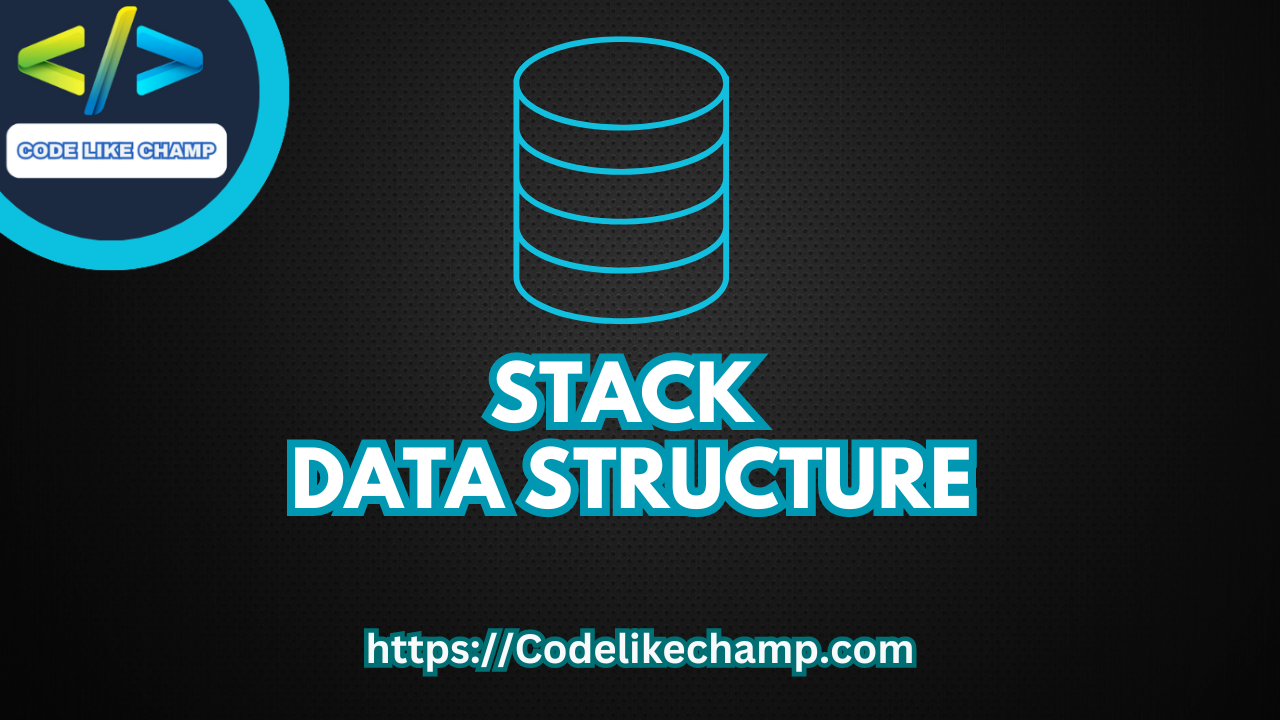
What is a Stack
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle, where the last element added to the stacks is the first one to be removed. It can be envisioned as a collection of elements stacked one on top of the other, akin to a stacks of plates. Elements can only be added or removed from the top of the stacks, making it a simple yet powerful abstraction for various computational tasks.
Why We Use Stacks in Programming
Stacks are widely used in programming for several reasons:
- Function Call Management: Stacks play a crucial role in managing function calls and maintaining their execution context, including local variables and return addresses.
- Expression Evaluation: Stacks are utilized in evaluating arithmetic expressions, postfix notation (also known as Reverse Polish Notation), and infix to postfix conversion.
- Undo/Redo Mechanisms: Stacks are employed in implementing undo and redo functionalities in applications, allowing users to revert or reapply changes sequentially.
- Backtracking Algorithms: Stacks facilitate backtracking algorithms, such as depth-first search (DFS), by storing the path or state transitions.
Characteristics of Stacks
- Last In, First Out (LIFO): Elements are added and removed from the stacks in a Last In, First Out manner, resembling a vertical structure where the last item added is the first one removed.
- Limited Access: Unlike arrays or linked lists, stacks support limited access operations, primarily restricted to push (addition) and pop (removal) operations at the top of the stacks.
- Stacks Pointer: Stacks typically maintain a pointer or index indicating the top element, facilitating efficient insertion and removal operations.
- Dynamic Size: Stacks can dynamically grow or shrink based on the number of elements pushed or popped, allowing for flexible memory allocation.
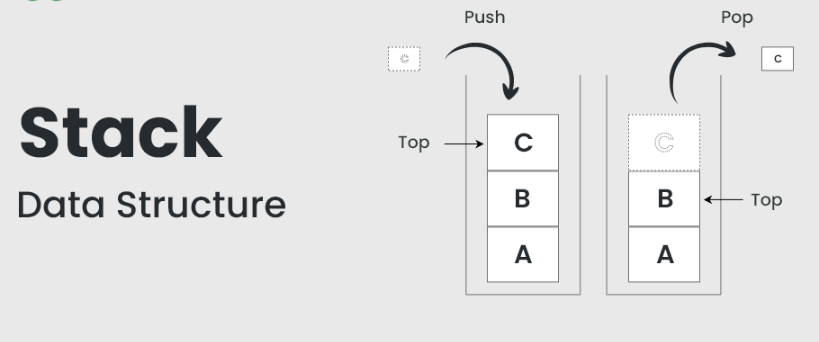
Types of Stack
- Array-based Stacks: In an array-based stacks, elements are stored in a contiguous block of memory, and the stacks pointer indicates the topmost element.
- Linked List-based Stacks: In a linked list-based stacks, elements are stored using a linked list data structure, providing dynamic memory allocation and efficient insertion and deletion operations.
- Dynamic Stacks: A dynamic stacks employs dynamic memory allocation techniques to resize the stack dynamically, accommodating varying data sizes.
Advantages of Stacks data structure
- Efficient Insertion and Removal: Stacks offer constant-time insertion and removal operations at the top, facilitating efficient stacks management.
- Function Call Management: Stacks simplify the management of function calls, supporting recursion, nested function calls, and maintaining execution contexts.
- Expression Evaluation: Stacks enable efficient evaluation of arithmetic expressions and postfix notation, simplifying complex calculations.
- Undo/Redo Functionality: Stacks facilitate the implementation of undo and redo functionalities, enhancing user interaction and workflow management.
Dis-Advantages of Stacks data structure
- Limited Access: Stacks provide limited access operations, restricting direct access to elements other than the topmost one.
- Dynamic Memory Management Overhead: Dynamic resizing of stacks may incur overhead due to memory reallocation and copying of elements.
- Stack Overflow: Uncontrolled recursion or excessive function calls may lead to stack overflow errors, exhausting available stack space.
- Lack of Random Access: Stacks do not support random access to elements, necessitating sequential traversal for accessing specific items.
Code Examples
Below are code examples demonstrating stack usage in various programming languages:
Stacks in C#
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { Stack<int> stack = new Stack<int>(); stack.Push(1); stack.Push(2); stack.Push(3); while (stack.Count > 0) { Console.Write(stack.Pop() + " "); } } }
Output
3 2 1
Stacks in in C
#include <stdio.h> #include <stdlib.h> #define MAX_SIZE 100 typedef struct { int items[MAX_SIZE]; int top; } Stack; void push(Stack *s, int value) { if (s->top == MAX_SIZE - 1) { printf("Stack Overflow\n"); return; } s->items[++(s->top)] = value; } int pop(Stack *s) { if (s->top == -1) { printf("Stack Underflow\n"); exit(1); } return s->items[(s->top)--]; } int main() { Stack s; s.top = -1; push(&s, 1); push(&s, 2); push(&s, 3); while (s.top > -1) { printf("%d ", pop(&s)); } return 0; }
Output
3 2 1
Stacks in C++
#include <iostream> #include <stack> int main() { std::stack<int> stack; stack.push(1); stack.push(2); stack.push(3); while (!stack.empty()) { std::cout << stack.top() << " "; stack.pop(); } return 0; }
Output
3 2 1
Stacks in Python
stack = [] stack.append(1) stack.append(2) stack.append(3) while stack: print(stack.pop(), end=" ")
Output
3 2 1
Stacks in PHP
<?php $stack = new SplStack(); $stack->push(1); $stack->push(2); $stack->push(3); while (!$stack->isEmpty()) { echo $stack->pop() . " "; } ?>
Output
3 2 1
Stacks in JAVA
import java.util.Stack; public class Main { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(1); stack.push(2); stack.push(3); while (!stack.isEmpty()) { System.out.print(stack.pop() + " "); } } }
Output
3 2 1
Stacks in JavaScript
let stack = []; stack.push(1); stack.push(2); stack.push(3); while (stack.length > 0) { console.log(stack.pop()); }
Output
3 2 1
Conclusion to Stacks Data Structure
In conclusion, stacks represent a vital data structure in the realm of computer science and programming, offering efficient storage and management of elements adhering to the Last In, First Out (LIFO) principle.
Despite their limitations, such as limited access and potential stacks overflow issues, stacks find widespread applications in diverse domains, ranging from function call management to expression evaluation and workflow automation. By mastering the concepts and applications of stacks, programmers can enhance their problem-solving skills and develop robust, efficient algorithms, contributing to the advancement of technology and innovation in the digital age.
For more visit my website Codelikechamp.com