Introduction to ArrayList
In the realm of programming, data structures play a pivotal role in organizing and managing data efficiently. Among the array-based data structures, ArrayList stands out as a versatile and widely-used container. In this article, we explore the nuances of ArrayList, from its definition to its applications, characteristics, types, and merits, as well as its demerits.
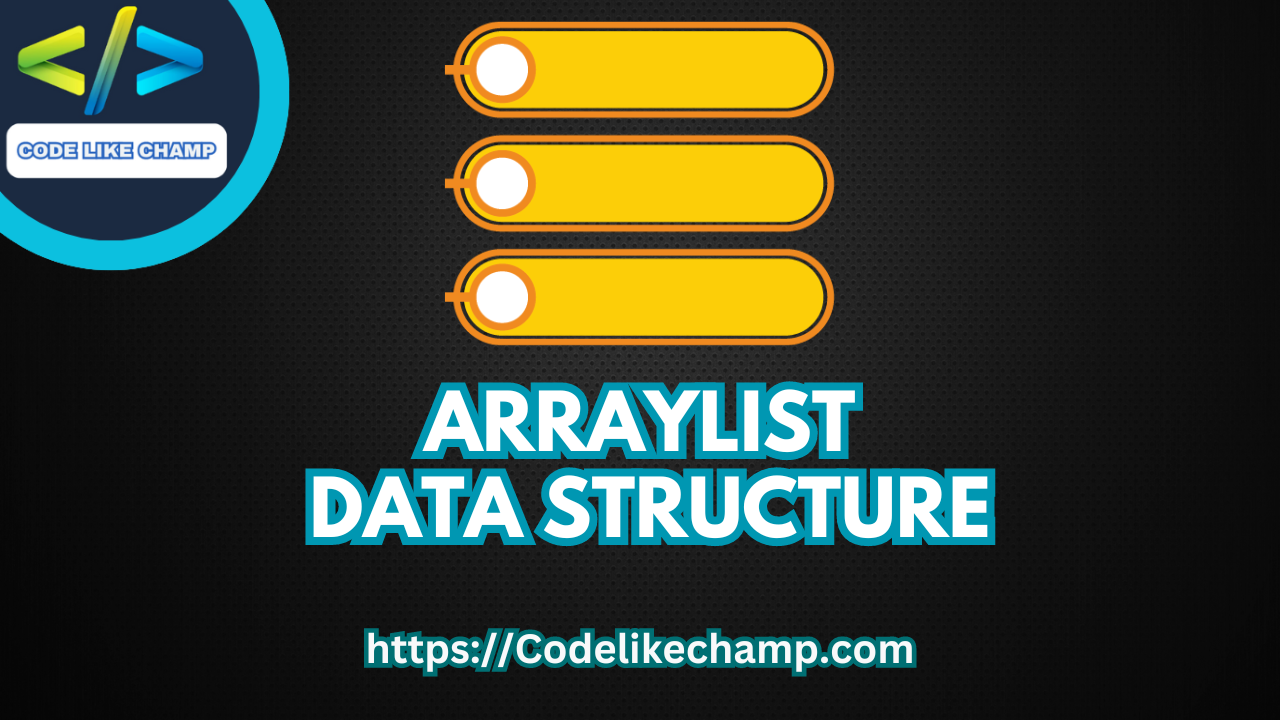
What is ArrayList
ArrayList is a dynamic array-based implementation of the List interface in many programming languages. It provides a resizable array to store elements and offers dynamic resizing capabilities, allowing for the addition and removal of elements. ArrayLists are widely used due to their flexibility, efficiency, and ease of use.
Why We Use ArrayLists in Programming
ArrayLists are utilized in programming for various reasons:
- Dynamic Size: ArrayLists automatically resize themselves to accommodate a varying number of elements, eliminating the need for manual memory management.
- Random Access: ArrayLists support efficient random access to elements using indices, enabling fast retrieval and manipulation of data.
- Versatility: ArrayLists can store elements of any data type, making them suitable for a wide range of applications.
- Standard Library Support: Most programming languages provide built-in support or standard libraries for ArrayLists, making them easy to use and integrate into applications.
Characteristics of ArrayLists
- Dynamic Resizing: ArrayLists dynamically resize themselves as elements are added or removed, ensuring efficient memory usage and avoiding unnecessary reallocations.
- Random Access: ArrayLists support efficient random access to elements using indices, facilitating fast retrieval and manipulation of data.
- Contiguous Memory: ArrayLists allocate memory in a contiguous block, leading to better cache locality and efficient memory usage compared to linked data structures.
- Standard Library Integration: ArrayLists are commonly included in standard libraries or provided as built-in data structures in most programming languages, simplifying their usage and integration.
Types of ArrayLists
- Standard ArrayLists: The standard ArrayList implementation provided by programming languages, typically offering a resizable array with basic functionalities.
- Specialized ArrayLists: Some programming languages offer specialized ArrayList implementations optimized for specific use cases, such as concurrent ArrayLists for multithreaded applications or synchronized ArrayLists for thread-safe operations.

Advantages of ArrayLists
- Dynamic Size: ArrayLists can grow or shrink dynamically, accommodating a variable number of elements without manual memory management.
- Random Access: ArrayLists support efficient random access to elements using indices, enabling fast retrieval and manipulation of data.
- Versatility: ArrayLists can store elements of any data type, making them suitable for a wide range of applications.
- Standard Library Support: Most programming languages provide built-in support or standard libraries for ArrayLists, making them easy to use and integrate into applications.
Dis-Advantages of ArrayLists
- Dynamic Resizing Overhead: Dynamic resizing operations, such as adding or removing elements beyond the allocated capacity, may incur overhead due to memory reallocation and copying of elements.
- Inefficient Insertions and Deletions: Inserting or deleting elements in the middle of an ArrayList may require shifting subsequent elements, resulting in linear time complexity and potential performance degradation.
- Memory Fragmentation: Frequent resizing operations and reallocations may lead to memory fragmentation, reducing overall memory efficiency and increasing the risk of memory leaks.
- Lack of Support for Non-contiguous Data: ArrayLists are limited to storing elements in a contiguous block of memory, making them unsuitable for scenarios requiring non-contiguous or linked data structures.
Code Examples of ArrayLists
Below are code examples demonstrating ArrayList usage in various programming languages:
ArrayLists in C#
using System; using System.Collections.Generic; class Program { static void Main() { // Create an ArrayList of integers List<int> arrayList = new List<int>(); // Add elements to the ArrayList arrayList.Add(1); arrayList.Add(2); arrayList.Add(3); // Display the elements of the ArrayList foreach (int num in arrayList) { Console.Write(num + " "); } Console.WriteLine(); } }
Output
1 2 3
ArrayLists in C
#include <stdio.h> int main() { // Create an ArrayList of integers int arrayList[3]; // Add elements to the ArrayList arrayList[0] = 1; arrayList[1] = 2; arrayList[2] = 3; // Display the elements of the ArrayList for (int i = 0; i < 3; i++) { printf("%d ", arrayList[i]); } printf("\n"); return 0; }
Output
1 2 3
ArrayLists in C++
#include <iostream> #include <vector> int main() { // Create an ArrayList of integers std::vector<int> arrayList; // Add elements to the ArrayList arrayList.push_back(1); arrayList.push_back(2); arrayList.push_back(3); // Display the elements of the ArrayList for (int num : arrayList) { std::cout << num << " "; } std::cout << std::endl; return 0; }
Output
1 2 3
ArrayLists in Python
# Create an ArrayList of integers arrayList = [] # Add elements to the ArrayList arrayList.append(1) arrayList.append(2) arrayList.append(3) # Display the elements of the ArrayList print(*arrayList)
Output
1 2 3
ArrayLists in PHP
<?php // Create an ArrayList of integers $arrayList = array(); // Add elements to the ArrayList $arrayList[] = 1; $arrayList[] = 2; $arrayList[] = 3; // Display the elements of the ArrayList echo implode(' ', $arrayList) . "\n"; ?>
Output
1 2 3
ArrayLists in JAVA
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { // Create an ArrayList of integers List<Integer> arrayList = new ArrayList<>(); // Add elements to the ArrayList arrayList.add(1); arrayList.add(2); arrayList.add(3); // Display the elements of the ArrayList for (int num : arrayList) { System.out.print(num + " "); } System.out.println(); } }
Output
1 2 3
ArrayLists in JavaScript
// Create an ArrayList of integers let arrayList = []; // Add elements to the ArrayList arrayList.push(1); arrayList.push(2); arrayList.push(3); // Display the elements of the ArrayList console.log(arrayList.join(' '));
Output
1 2 3
Conclusion
In conclusion, ArrayLists serve as versatile and efficient containers for managing dynamic arrays in programming. They offer dynamic resizing, efficient random access, and standard library support, making them indispensable tools for developers across various domains. Despite some limitations such as dynamic resizing overhead and inefficient insertions/deletions, ArrayLists remain a popular choice for storing and manipulating data in modern software applications. By understanding the characteristics, types, merits, and demerits of ArrayLists, programmers can harness the full potential of this powerful data structure to develop robust and scalable solutions, contributing to the advancement of technology and innovation in the digital age.
For more visit my website Codelikechamp.com