Introduction to Vector data structure
In the realm of programming and software development, data structures play a fundamental role in organizing and managing data efficiently. Among the plethora of data structures, the vector stands out as a versatile container for dynamic arrays. In this comprehensive article, we delve into the intricacies of the vector data structure , examining its definition, utility, characteristics, types, merits, and demerits.
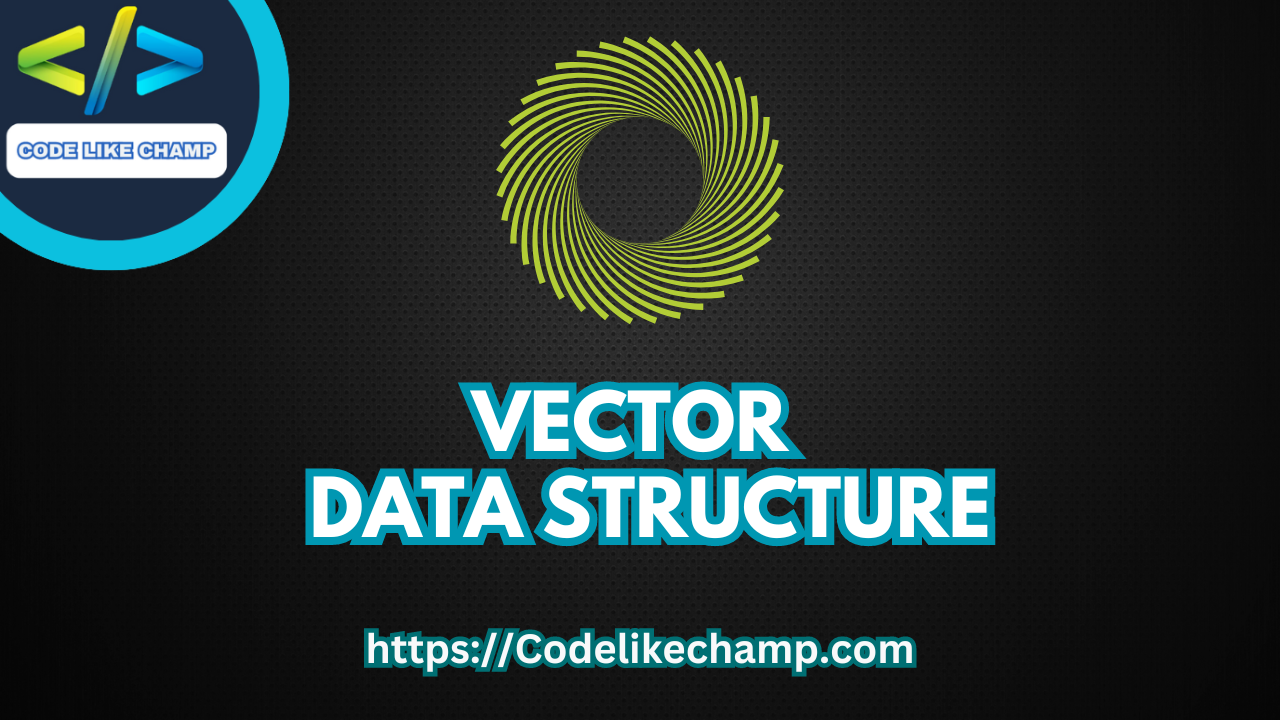
What is Vector Data Structures
A vector, also known as a dynamic array, is a linear data structure that dynamically adjusts its size to accommodate elements. It provides a contiguous block of memory to store elements and allows for efficient random access to elements using indices. Vectors are typically implemented as resizable arrays, enabling the addition and removal of elements with amortized constant time complexity.
Why We Use Vector Data Structures in Programming
Vector data structures are widely used in programming for several reasons:
- Dynamic Size: Vectors dynamically resize themselves to accommodate a varying number of elements, making them suitable for situations where the number of elements is not known in advance.
- Random Access: Vectors allow for efficient random access to elements using indices, enabling fast retrieval and manipulation of data.
- Memory Efficiency: Vectors allocate memory in a contiguous block, leading to better cache locality and efficient memory usage compared to linked data structures.
- Standard Library Support: Most programming languages provide built-in support or standard libraries for vectors, making them easy to use and integrate into applications.
Characteristics of Vector Data Structures
- Contiguous Memory: Vectors allocate memory in a contiguous block, allowing for efficient random access to elements using indices.
- Dynamic Resizing: Vectors dynamically resize themselves as elements are added or removed, ensuring efficient memory usage and avoiding unnecessary reallocations.
- Random Access: Vectors support efficient random access to elements using indices, enabling fast retrieval and manipulation of data.
- Amortized Constant Time Complexity: Vectors offer amortized constant time complexity for adding or removing elements at the end of the container, making them efficient for dynamic arrays.
Types of Vector Data Structures
- Standard Vector: The standard vector, available in most programming languages, dynamically resizes itself to accommodate elements and provides efficient random access.
- Specialized Vectors: Some programming languages offer specialized vector implementations optimized for specific use cases, such as bit vectors for storing binary data or sparse vectors for handling large, mostly empty datasets.
Merit and Demerits of Vector Data Structures:
Merits:
- Dynamic Size: Vectors can grow or shrink dynamically, allowing for flexible storage of elements without the need for manual memory management.
- Random Access: Vectors support efficient random access to elements using indices, facilitating fast retrieval and manipulation of data.
- Memory Efficiency: Vectors allocate memory in a contiguous block, leading to better cache locality and efficient memory usage compared to linked data structures.
- Standard Library Support: Most programming languages provide built-in support or standard libraries for vectors, making them easy to use and integrate into applications.
Demerits:
- Dynamic Resizing Overhead: Dynamic resizing operations, such as adding or removing elements beyond the allocated capacity, may incur overhead due to memory reallocation and copying of elements.
- Inefficient Insertions and Deletions: Inserting or deleting elements in the middle of a vector may require shifting subsequent elements, resulting in linear time complexity and potential performance degradation.
- Memory Fragmentation: Frequent resizing operations and reallocations may lead to memory fragmentation, reducing overall memory efficiency and increasing the risk of memory leaks.
- Lack of Support for Non-contiguous Data: Vectors are limited to storing elements in a contiguous block of memory, making them unsuitable for scenarios requiring non-contiguous or linked data structures.
Code Examples of Vector data structures
Below are code examples demonstrating vector usage in various programming languages:
Vector data structures in C++
#include <iostream> #include <vector> int main() { // Create a vector of integers std::vector<int> vec = {1, 2, 3, 4, 5}; // Add an element to the vector vec.push_back(6); // Display the elements of the vector for (int num : vec) { std::cout << num << " "; } std::cout << std::endl; return 0; }
Output
1 2 3 4 5 6
Vector data structures in Python
# Create a list of integers vec = [1, 2, 3, 4, 5] # Add an element to the list vec.append(6) # Display the elements of the list print(*vec)
Output
1 2 3 4 5 6
Vector data structures in JAVA
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { // Create a list of integers List<Integer> list = new ArrayList<>(); list.add(1); list.add(2); list.add(3); list.add(4); list.add(5); // Add an element to the list list.add(6); // Display the elements of the list for (int num : list) { System.out.print(num + " "); } System.out.println(); } }
Output
1 2 3 4 5 6
Vector data structures in JavaScript
// Create an array of integers let vec = [1, 2, 3, 4, 5]; // Add an element to the array vec.push(6); // Display the elements of the array console.log(vec.join(' '));
Output
1 2 3 4 5 6
These code examples demonstrate creating a vector (or equivalent data structure) of integers, adding an element to it, and then displaying all the elements. Each language-specific implementation achieves the same functionality using their respective syntax and libraries.
Conclusion
In conclusion, the vector data structure serves as a versatile container for dynamic arrays, offering dynamic resizing, efficient random access, and memory efficiency. Despite some limitations such as dynamic resizing overhead and inefficient insertions/deletions, vectors remain a popular choice for storing and manipulating data in various programming languages. By understanding the characteristics, types, merits, and demerits of vectors, programmers can leverage this powerful data structure to develop efficient and scalable applications, contributing to the advancement of technology and innovation in the digital age. So if you face reading or understanding this article just contact me with any hesitation.
For more visit my website Codelikechamp.com