Introduction to Binary Tree
In the realm of data structures, a Binary Tree stands as one of the foundational structures, offering efficient storage and retrieval capabilities. In this article, we delve into the intricacies of Binary Trees, exploring their characteristics, types, advantages, and disadvantages.

What is Binary Trees
A Binary Tree is a hierarchical data structure composed of nodes, where each node has at most two children, referred to as the left child and the right child. The topmost node of the tree is called the root node, while nodes with no children are known as leaf nodes.
Why We Use Binary Trees in Data Structure
Binary Trees are widely used in data structures due to their versatility and efficiency in various operations such as searching, insertion, deletion, and traversal. Their hierarchical nature allows for organized storage of data, making them ideal for applications like database indexing, expression parsing, and hierarchical data representation.
Characteristics of Binary Trees
- Hierarchical Structure: Nodes are organized in a hierarchical manner, with each node having at most two children.
- Root Node: The topmost node of the tree is called the root node, serving as the entry point for accessing other nodes.
- Binary Nature: Each node can have at most two children, distinguishing Binary Trees from other tree structures.
- Recursive Definition: Binary Trees can be defined recursively, with each subtree also being a Binary Tree.
- Balanced vs. Unbalanced: Binary Trees can be balanced, ensuring relatively equal height for subtrees, or unbalanced, leading to skewed structures with significant height differences.
Types of Binary Trees
- Full Binary Trees: A Binary Tree where each node has either zero or two children.
- Complete Binary Trees: A Binary Tree in which all levels are completely filled except possibly for the last level, which is filled from left to right.
- Perfect Binary Trees: A Binary Tree where all internal nodes have exactly two children and all leaf nodes are at the same level.
- Balanced Binary Trees: A Binary Tree in which the height of the left and right subtrees of any node differ by at most one.
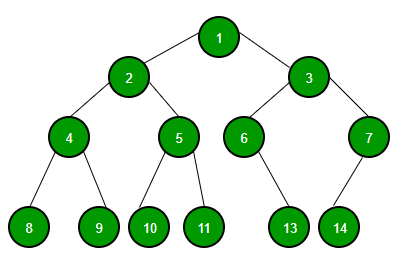
Advantages of Binary Trees
- Efficient Searching: Binary Trees facilitate efficient searching operations, especially in sorted trees like Binary Search Trees (BST).
- Space Efficiency: Binary Trees optimize space utilization by organizing data in a hierarchical manner, reducing storage overhead.
- Flexibility: Binary Trees can be dynamically modified through operations like insertion and deletion, accommodating changing data requirements.
- Ordered Access: In Binary Search Trees, elements are stored in sorted order, enabling quick retrieval and range-based operations.
Disadvantages of Binary Trees
- Unbalanced Structures: Without proper balancing mechanisms, Binary Trees can degrade into unbalanced structures, leading to inefficient operations.
- Complexity: Implementing and managing Binary Trees, especially balanced variants, can be complex and require careful consideration of algorithms and data structures.
- Limited Functionality: While efficient for certain operations like searching and sorting, Binary Trees may not be suitable for all types of data and applications, requiring alternative structures for specific use cases.
Code Examples in Various Languages
Below are code examples demonstrating the implementation of a Binary Trees in different programming languages:
Binary Trees in C#
using System; public class Node { public int data; public Node left, right; public Node(int item) { data = item; left = right = null; } } public class BinaryTree { Node root; public void PrintTree(Node node) { if (node == null) return; // Traverse left subtree PrintTree(node.left); // Print current node's data Console.Write(node.data + " "); // Traverse right subtree PrintTree(node.right); } public static void Main(string[] args) { BinaryTree tree = new BinaryTree(); // Create sample binary tree tree.root = new Node(1); tree.root.left = new Node(2); tree.root.right = new Node(3); tree.root.left.left = new Node(4); tree.root.left.right = new Node(5); Console.WriteLine("Binary Tree:"); tree.PrintTree(tree.root); } }
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Trees in C
#include <stdio.h> #include <stdlib.h> struct Node { int data; struct Node* left; struct Node* right; }; struct Node* createNode(int data) { struct Node* newNode = (struct Node*)malloc(sizeof(struct Node)); newNode->data = data; newNode->left = NULL; newNode->right = NULL; return newNode; } void printTree(struct Node* node) { if (node == NULL) return; // Traverse left subtree printTree(node->left); // Print current node's data printf("%d ", node->data); // Traverse right subtree printTree(node->right); } int main() { struct Node* root = createNode(1); root->left = createNode(2); root->right = createNode(3); root->left->left = createNode(4); root->left->right = createNode(5); printf("Binary Tree:\n"); printTree(root); return 0; }
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Trees in C++
#include <iostream> using namespace std; class Node { public: int data; Node* left; Node* right; Node(int item) { data = item; left = right = NULL; } }; void printTree(Node* node) { if (node == NULL) return; // Traverse left subtree printTree(node->left); // Print current node's data cout << node->data << " "; // Traverse right subtree printTree(node->right); } int main() { Node* root = new Node(1); root->left = new Node(2); root->right = new Node(3); root->left->left = new Node(4); root->left->right = new Node(5); cout << "Binary Tree:" << endl; printTree(root); return 0; }
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Trees in Python
class Node: def __init__(self, data): self.data = data self.left = None self.right = None def print_tree(node): if node is None: return # Traverse left subtree print_tree(node.left) # Print current node's data print(node.data, end=" ") # Traverse right subtree print_tree(node.right) if __name__ == "__main__": # Create sample binary tree root = Node(1) root.left = Node(2) root.right = Node(3) root.left.left = Node(4) root.left.right = Node(5) print("Binary Tree:") print_tree(root)
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Trees in PHP
<?php class Node { public $data; public $left; public $right; public function __construct($item) { $this->data = $item; $this->left = null; $this->right = null; } } function printTree($node) { if ($node == null) return; // Traverse left subtree printTree($node->left); // Print current node's data echo $node->data . " "; // Traverse right subtree printTree($node->right); } // Create sample binary tree $root = new Node(1); $root->left = new Node(2); $root->right = new Node(3); $root->left->left = new Node(4); $root->left->right = new Node(5); echo "Binary Tree:\n"; printTree($root); ?>
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Trees in JAVA
class Node { int data; Node left, right; public Node(int item) { data = item; left = right = null; } } public class BinaryTree { Node root; void printTree(Node node) { if (node == null) return; // Traverse left subtree printTree(node.left); // Print current node's data System.out.print(node.data + " "); // Traverse right subtree printTree(node.right); } public static void main(String[] args) { BinaryTree tree = new BinaryTree(); // Create sample binary tree tree.root = new Node(1); tree.root.left = new Node(2); tree.root.right = new Node(3); tree.root.left.left = new Node(4); tree.root.left.right = new Node(5); System.out.println("Binary Tree:"); tree.printTree(tree.root); } }
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Binary Tree in JavaScript
class Node { constructor(data) { this.data = data; this.left = null; this.right = null; } } function printTree(node) { if (node === null) return; // Traverse left subtree printTree(node.left); // Print current node's data process.stdout.write(node.data + " "); // Traverse right subtree printTree(node.right); } // Create sample binary tree let root = new Node(1); root.left = new Node(2); root.right = new Node(3); root.left.left = new Node(4); root.left.right = new Node(5); console.log("Binary Tree:"); printTree(root);
Output
Binary Tree: 4 2 5 1 3
Explanation: The code creates a binary tree with sample nodes and then traverses the tree in an inorder manner(LEFT-NODE THEN ROOT THEN RIGHT NODE), printing the data of each node.
Conclusion
In conclusion, Binary Search Trees serve as essential tools in the domain of data structures, providing efficient storage, retrieval, and ordering capabilities. By understanding their characteristics, types, advantages, and disadvantages, developers can leverage the power of Binary Search Trees to design and implement optimized solutions for various applications. With their balanced performance and dynamic operations, Binary Search Trees continue to play a vital role in software development and algorithmic problem-solving.
For more visit my website Codelikechamp.com
If you face any issue regarding this topic or any other topic please ask or contact me without any hesitation.
For more visit my website Codelikechamp.com
This entry is unbelievable. The brilliant substance reveals the distributer’s interest. I’m stunned and anticipate more such astonishing sections.
you will see my brother this is just beginning i will prove that the content i produced is far better than any website
Hi everybody, here every person is sharing these familiarity, so it’s fastidious to read this website, and I used to pay a visit this weblog daily.
hey buddy what problem you are facing please tell me how can i help you ????
Asking questions are truly good thing if you are not understanding anything fully, however this
post gives fastidious understanding even.
thanks for your compliment if you want any help just contact and please share with your relative